
dev-resources.site
for different kinds of informations.
Php Base64 encode/decode – best practices and use cases
Base64 encoding in PHP is a simple yet powerful tool for transmitting binary data as text. Here's what you need to know:
- What It Does: Converts binary data into a safe text format using a 64-character set, ideal for text-based protocols.
- Common Uses: Encoding email attachments, embedding images in JSON/HTML, and storing binary data in text-only databases.
- Key Limitations: Base64 is not encryption; always pair it with secure methods for sensitive data.
- Best Practices: Validate input, handle errors with strict mode, and avoid misuse for large files or database storage.
Quick Tip: Use base64url_encode()
for web-safe encoding in URLs or JSON. For large files, process data in chunks or streams to optimize memory usage.
Keep reading for practical examples, error handling, and performance tips!
Base64 Encode & Decode in PHP
How Base64 Encoding and Decoding Works in PHP
PHP comes with built-in functions to handle Base64 encoding and decoding, making it easier to securely transmit data over text-based protocols. Let's dive into how these functions work and how to use them effectively.
Encoding with base64_encode()
The base64_encode()
function converts binary data into Base64 text, which is safe for transmission over text-based systems. Here's an example:
// Encode a simple string to Base64
$original_string = "Hello, world!";
$encoded_string = base64_encode($original_string);
echo $encoded_string; // Output: SGVsbG8sIHdvcmxkIQ==
// Encode binary data, such as an image
$image_data = file_get_contents('logo.png');
$base64_image = base64_encode($image_data);
This function is particularly useful for encoding files or binary data into a text-friendly format.
Decoding with base64_decode()
The base64_decode()
function reverses the encoding process, converting Base64 text back to its original binary form. Using strict mode ensures that only valid Base64 data is processed:
// Decode a Base64 string
$encoded_string = "SGVsbG8sIHdvcmxkIQ==";
$decoded_string = base64_decode($encoded_string);
echo $decoded_string; // Output: Hello, world!
// Strict mode example for validation
$decoded_strict = base64_decode($encoded_string, true);
if ($decoded_strict === false) {
echo "Invalid Base64 data detected";
} else {
echo "Successfully decoded: " . $decoded_strict;
}
Strict mode is a great way to prevent errors caused by malformed or invalid Base64 input.
Handling Base64 Safely
To avoid common pitfalls, consider these robust approaches for encoding and decoding:
// Encode data with validation
function safeBase64Encode($data) {
if (empty($data)) {
throw new InvalidArgumentException("Input data cannot be empty");
}
return base64_encode($data);
}
// Decode data with error handling
function safeBase64Decode($encodedData) {
try {
$decoded = base64_decode($encodedData, true); // Use strict mode
if ($decoded === false) {
throw new Exception("Invalid Base64 data");
}
return $decoded;
} catch (Exception $e) {
error_log("Base64 decoding error: " . $e->getMessage());
return false;
}
}
Best Practices for PHP Base64 Encoding
Input Data Validation and Sanitization
When working with Base64 encoding, validating and sanitizing your input data is critical. Here's a straightforward way to handle this:
function validateAndEncode($data) {
if (empty($data) || !is_string($data)) {
throw new InvalidArgumentException("Invalid input data");
}
$sanitized = filter_var($data, FILTER_SANITIZE_STRING);
$encoded = base64_encode($sanitized);
if ($encoded === false) {
throw new RuntimeException("Encoding failed");
}
return $encoded;
}
For decoding, ensure you use strict mode to maintain data integrity:
function validateAndDecode($encodedData) {
if (!preg_match('/^[A-Za-z0-9+\/]+={0,2}$/', $encodedData)) {
throw new InvalidArgumentException("Invalid Base64 format");
}
$decoded = base64_decode($encodedData, true);
if ($decoded === false) {
throw new RuntimeException("Decoding failed");
}
return $decoded;
}
Performance and Resource Optimization
Base64 encoding can be resource-intensive, especially for larger data. Here's how to handle different scenarios efficiently:
- Data under 1MB: Direct encoding works well.
- Data between 1MB and 10MB: Process in chunks to save memory.
- Data over 10MB: Use stream processing to minimize memory usage.
For example, encoding large files in chunks:
function encodeInChunks($filePath, $chunkSize = 1024 * 1024) {
$fileHandle = fopen($filePath, 'rb');
$encoded = '';
while (!feof($fileHandle)) {
$chunk = fread($fileHandle, $chunkSize);
$encoded .= base64_encode($chunk);
}
fclose($fileHandle);
return $encoded;
}
This approach helps prevent memory overload while handling large files effectively.
Avoiding Misuse of Base64 Encoding
Base64 isn't the right choice for every situation. Misusing it can lead to inefficiencies. For instance:
// Avoid storing large files directly in the database
$imageData = base64_encode(file_get_contents('large_image.jpg'));
$db->query("INSERT INTO images (data) VALUES ('$imageData')");
// Instead, store the file path and use the filesystem
$filePath = '/uploads/' . uniqid() . '.jpg';
move_uploaded_file($_FILES['image']['tmp_name'], $filePath);
$db->query("INSERT INTO images (file_path) VALUES ('$filePath')");
Understanding when to use Base64 and when to explore other options can save both time and resources.
Practical Applications of PHP Base64 Encoding
Secure Data Transmission
Base64 encoding is commonly used to encode API keys or tokens for transmission. However, it's important to note that Base64 itself doesn't provide encryption or security. To ensure safety, always combine it with encryption protocols like HTTPS or token-based authentication.
function prepareApiRequest($apiKey, $payload) {
$credentials = base64_encode($apiKey);
$headers = [
'Authorization: Bearer ' . $credentials,
'Content-Type: application/json'
];
return [
'headers' => $headers,
'payload' => json_encode($payload)
];
}
Aside from secure transmission, Base64 encoding is also frequently used for embedding media directly into web content, making it a versatile tool.
Embedding Media in JSON or HTML
Base64 is a handy option for embedding small assets (less than 50KB) directly into JSON or HTML. This approach reduces the need for additional network requests. However, it does increase the overall payload size, making it less suitable for larger files.
function embedImageInResponse($imagePath, $maxSize = 1048576) {
if (!file_exists($imagePath)) {
throw new RuntimeException('Image not found');
}
$fileSize = filesize($imagePath);
if ($fileSize > $maxSize) {
throw new RuntimeException('Image exceeds maximum size limit');
}
$mimeType = mime_content_type($imagePath);
$imageData = base64_encode(file_get_contents($imagePath));
return [
'data_uri' => "data:$mimeType;base64,$imageData",
'size' => $fileSize
];
}
While Base64 works well for small files, it can lead to increased payload size and memory usage when used with larger files, so use it wisely.
Email Attachments and MIME
Base64 encoding plays a key role in ensuring email attachments are properly formatted for reliable delivery across different email clients. The chunk_split function is often used to break the encoded content into smaller lines, complying with MIME standards.
function attachFileToEmail($filePath) {
$content = chunk_split(base64_encode(file_get_contents($filePath)));
$filename = basename($filePath);
$headers = [
"Content-Type: application/octet-stream; name=\"$filename\"",
"Content-Transfer-Encoding: base64",
"Content-Disposition: attachment; filename=\"$filename\""
];
return [
'content' => $content,
'headers' => implode("\r\n", $headers)
];
}
Using Inspector for Debugging Base64 in PHP
Inspector offers tools to debug Base64 operations in PHP, making it easier to pinpoint and fix encoding issues that might compromise data during transmission or processing.
About Inspector
Inspector is a real-time monitoring tool designed for PHP frameworks. It provides insights into the executions of code blocks and overall application behavior. It tracks both synchronous and asynchronous Base64 processes, simplifying debugging without requiring a complicated setup.
Integrating Inspector in your PHP app
1) Install the Package
Run the following command to add Inspector to your project:
composer require inspector-apm/inspector-php
Configure the Integration
Set up the configuration file to enable monitoring:
// config/inspector.php
return [
'ingestion_key' => 'your-inspector-key',
];
Monitor Base64 Operations
Use Inspector to track Base64 encoding and handle exceptions:
use Inspector\Inspector;
use Inspector\Models\Segment;
$inspector = new Inspector(
new Configuration(config('inspector')['ingestion_key'])
);
try {
$data = $inspector->addSegment(function (Segment $segment) {
$encoded = \base64_encode($data);
$segment->addContext('info', [
'type' => 'base64',
'data' => \strlen($encoded)
]);
return $encoded;
}, 'base64', 'encode');
} catch (\Exception $e) {
$inspector->reportException($e);
}
Inspector provides a free tier for smaller projects, with paid options for more advanced needs. Its real-time insights can help you quickly address encoding issues, ensuring smooth operation and reliable data handling for your users.
Conclusion and Key Points
To ensure smooth Base64 operations in PHP, keep these core practices in mind:
- Security: Always validate and sanitize inputs to block injection attacks.
- Performance: Handle large files in chunks and use streaming for resource-heavy tasks.
- Error Handling: Enable strict mode to catch potential issues early.
- Monitoring: Use tools like Inspector for real-time debugging and tracking.
By following these guidelines, you'll be well-prepared to use Base64 encoding effectively in practical applications.
Final Advice
Balancing security, performance, and error handling is key to using Base64 encoding efficiently in PHP. Stick to scenarios where Base64 is genuinely useful, such as:
- Embedding binary data into JSON payloads.
- Managing email attachments via MIME.
- Safely transmitting data in URLs with Base64URL.
- Avoid using Base64 for tasks it wasn’t designed for, like securing sensitive data. Misusing it can lead to vulnerabilities and reduced performance.
For ongoing monitoring and maintenance:
- Utilize debugging tools like Inspector to track operations in real time.
- Keep an eye on Base64 processes in both synchronous and asynchronous workflows.
- Validate all inputs and handle errors promptly to ensure reliable Base64 operations.
Related posts
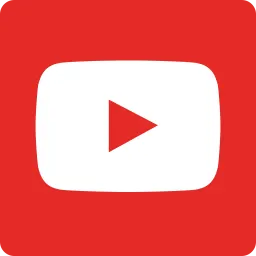
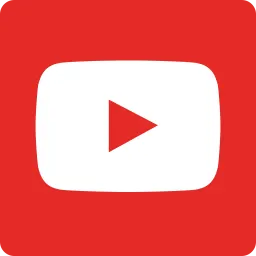
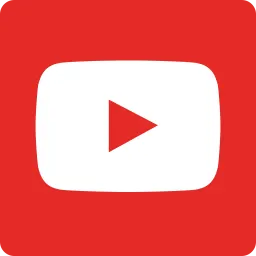
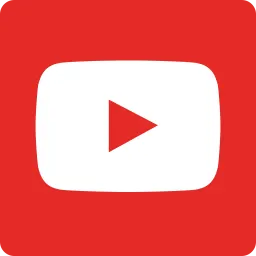
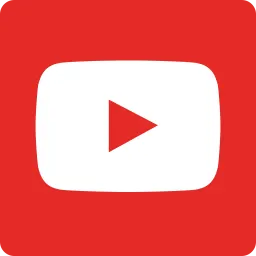
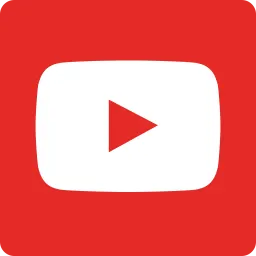
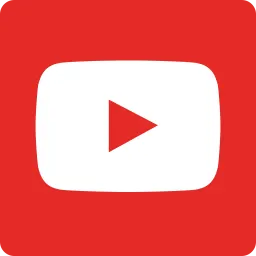
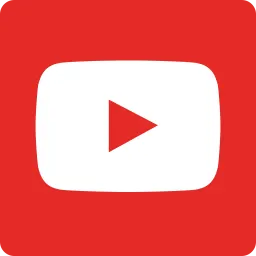
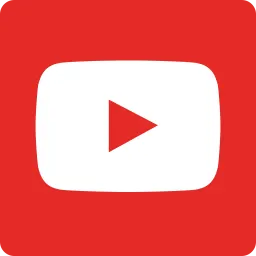
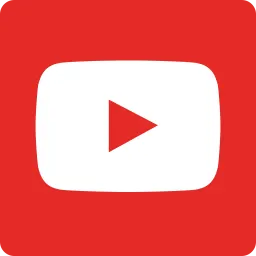
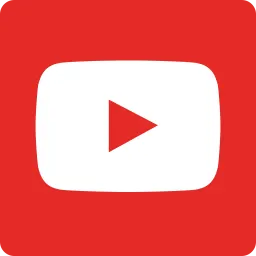
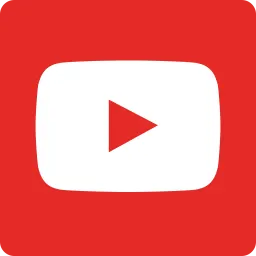
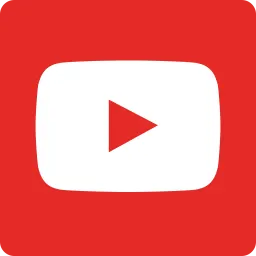
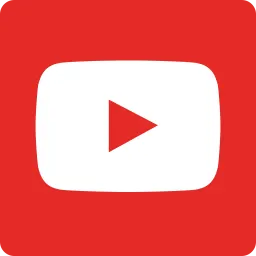
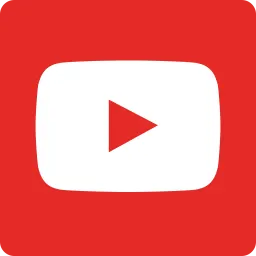
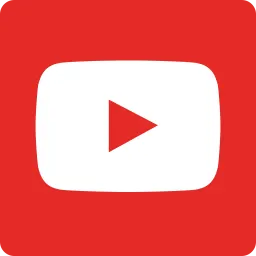
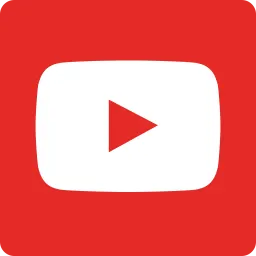
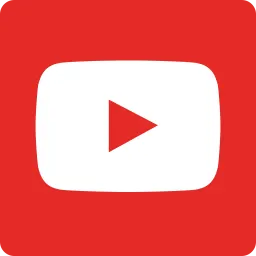
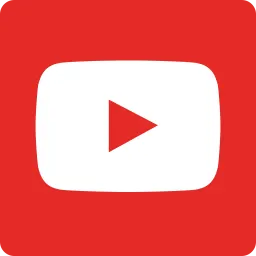
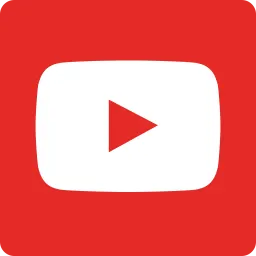
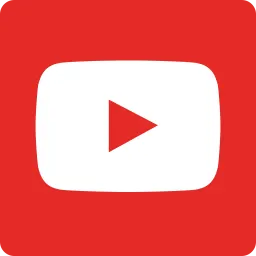
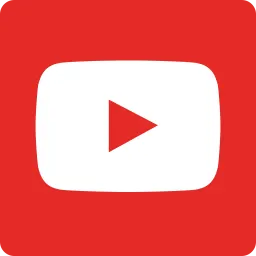
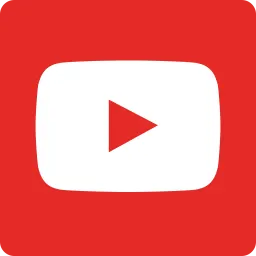
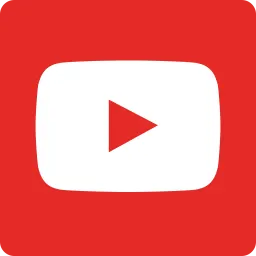
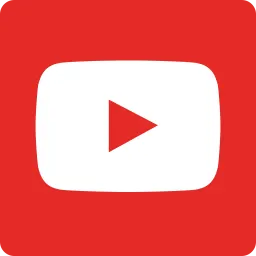
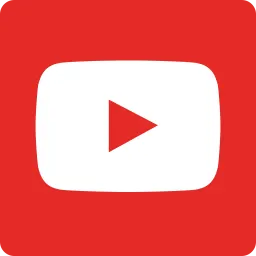
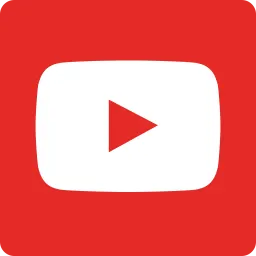
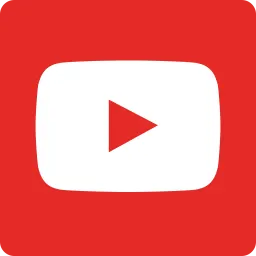
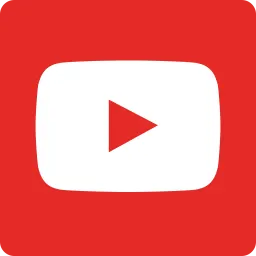
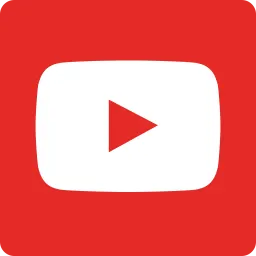
Featured ones: