
dev-resources.site
for different kinds of informations.
Response Handling Best Practices
Effective response handling is crucial in API design to ensure consistency, scalability, and a seamless experience for users. By adopting these best practices, you can enhance the quality of your APIs, irrespective of the programming language or framework used.
Key Best Practices
-
Use a Standardized Response Format:
- Establish a consistent structure for all API responses.
- Include fields such as
status
,message
, anddata
to make responses easy to interpret.
-
Paginate Results for Large Data Sets:
- Avoid returning excessively large datasets in a single response.
- Use pagination to limit the amount of data returned per request and include metadata such as
current_page
,total_items
, anditems_per_page
.
-
Use Clear and Meaningful Status Codes:
- Utilize standard HTTP status codes to indicate the outcome of a request.
- Ensure the status codes align with the type of response (e.g.,
200
for success,404
for not found, and500
for server errors).
-
Provide Descriptive Error Messages:
- Return meaningful error messages that help users understand what went wrong and how to fix it.
- Avoid exposing sensitive information in error details.
-
Include Resource Transformation:
- Transform data into a user-friendly format to prevent exposing internal implementation details.
- Ensure responses are concise, relevant, and easy to understand.
Standardized Response Structure
A standardized response structure helps users of your API quickly understand the data being returned. Here’s an example of a generalized structure:
Success Response:
{
"status": "success",
"message": "Product records fetched successfully.",
"data": {
"current_page": 1,
"data": [
{
"id": 1,
"item_id": 101,
"unit_of_measure": "kg",
"days_of_stock": 15,
"expected_stockout_date": "2025-01-30",
"recommended_order_date": "2025-01-25",
...
}
],
"first_page_url": "http://example.com/api/v1/logistix/products?page=1",
"from": 1,
"last_page": 10,
...
}
}
Error Response:
{
"status": "error",
"message": "Product details not found.",
"data": null
}
Implementing Pagination
For APIs returning large datasets, pagination is essential to:
- Improve performance by limiting the amount of data sent in each response.
- Provide a better user experience by organizing data into manageable chunks.
Include metadata in the response to guide users:
Pagination Metadata:
{
"current_page": 1,
"items_per_page": 10,
"total_items": 100,
"total_pages": 10
}
Error Handling Guidelines
-
Validation Errors:
- Clearly communicate which fields caused the error and why.
- Example:
"The 'email' field is required and must be a valid email address."
-
Authorization Errors:
- Use status codes like
401
(Unauthorized) or403
(Forbidden). - Avoid providing sensitive information in the response.
- Use status codes like
-
Server Errors:
- Return a generic message for unexpected issues, such as "An error occurred on the server. Please try again later."
Benefits of Adopting These Practices
-
Consistency:
- Standardized responses make it easier for developers to consume APIs and handle responses programmatically.
-
Scalability:
- Pagination and structured error handling ensure the API performs well even as data grows.
-
Improved User Experience:
- Clear error messages and well-structured responses enhance usability and reduce friction.
-
Maintainability:
- Following best practices makes your API easier to debug, extend, and support.
By adhering to these response handling best practices, you can deliver robust, reliable, and user-friendly APIs that are well-suited for diverse use cases and clients.
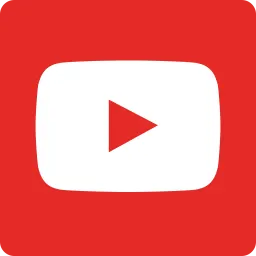
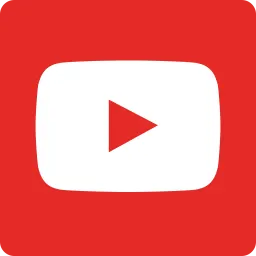
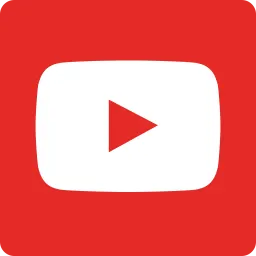
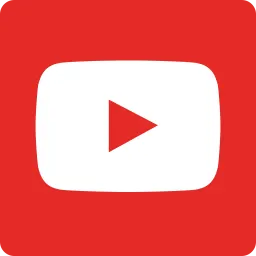
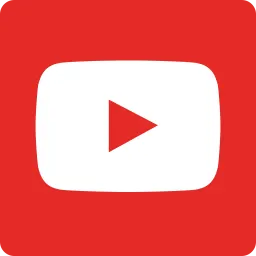
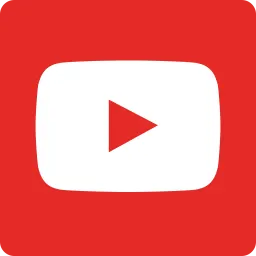
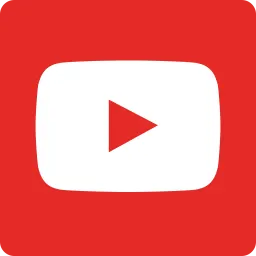
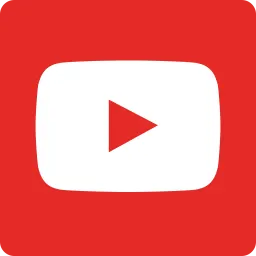
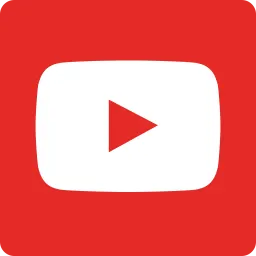
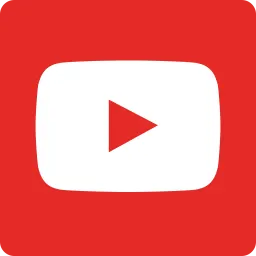
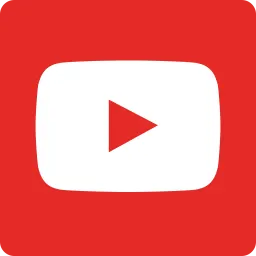
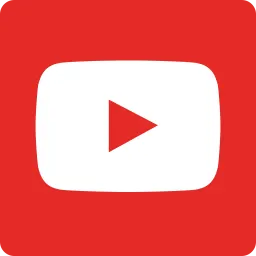
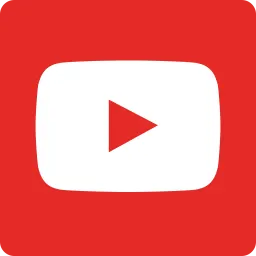
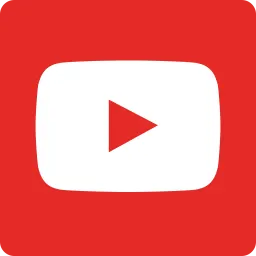
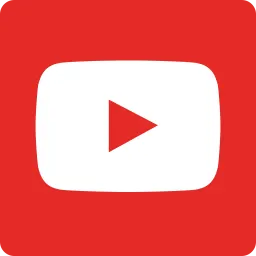
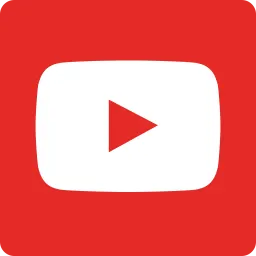
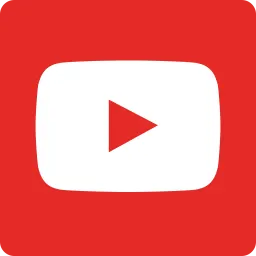
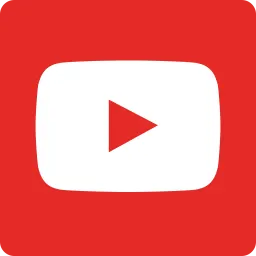
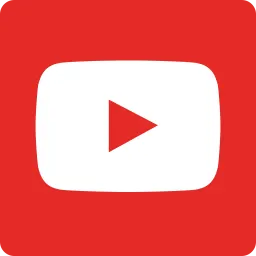
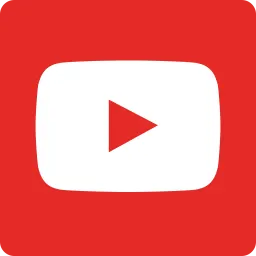
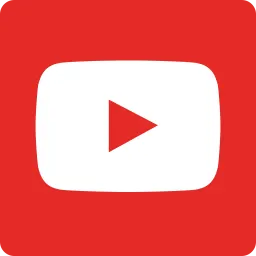
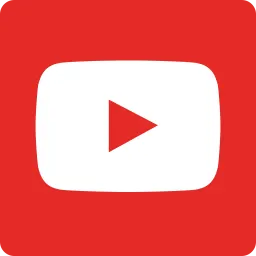
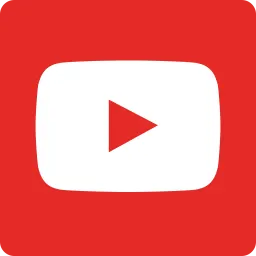
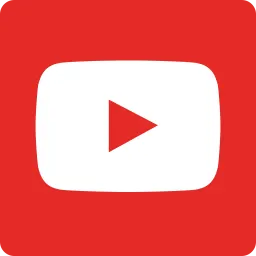
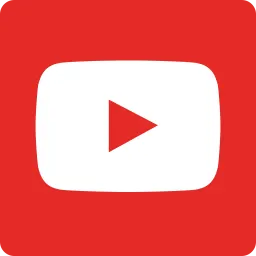
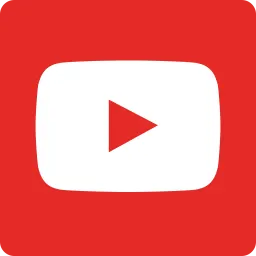
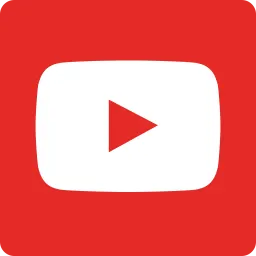
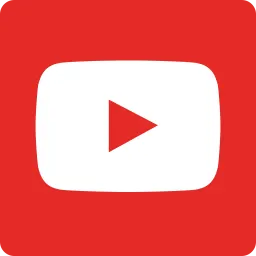
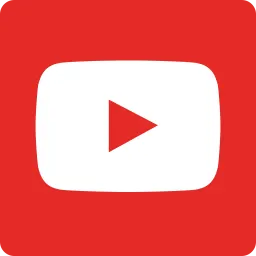
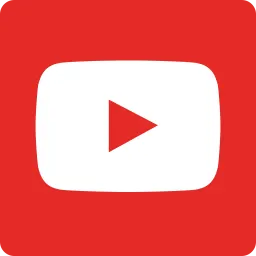
Featured ones: