
dev-resources.site
for different kinds of informations.
Understanding Observers in JavaScript: A Comprehensive Guide
Observers are an essential pattern in JavaScript, enabling developers to monitor and respond to changes in objects, events, or data streams. They provide the backbone for building dynamic, interactive applications. In this blog post, weโll dive deep into the types of observers in JavaScript, complete with detailed explanations and practical examples to help you master their usage.
1. Event Observers (Event Listeners)
Event listeners are the most commonly used observers in JavaScript. They monitor and respond to events such as user interactions (clicks, keypresses, or mouse movements).
Code Example:
// Select a button element
const button = document.querySelector('button');
// Attach an event listener to observe clicks
button.addEventListener('click', (event) => {
// Log when the button is clicked
console.log('Button clicked!', event);
});
// Add another listener for mouseover events
button.addEventListener('mouseover', () => {
console.log('Mouse is over the button!');
});
Use Cases:
- Handling form submissions.
- Observing drag-and-drop interactions.
- Tracking navigation clicks.
- Implementing keyboard shortcuts for improved accessibility.
- Adding hover or mouseover effects for dynamic UI feedback.
2. Mutation Observers
Mutation observers monitor changes to the DOM, such as added, removed, or modified nodes. This makes them invaluable for applications where the DOM changes dynamically.
Code Example:
// Select the element to observe
const targetNode = document.querySelector('#target');
// Create a MutationObserver instance
const observer = new MutationObserver((mutationsList) => {
mutationsList.forEach((mutation) => {
console.log('Mutation detected:', mutation);
});
});
// Configuration options for observing
const config = {
childList: true, // Watch for addition or removal of child nodes
attributes: true, // Watch for changes to attributes
subtree: true, // Watch for changes in descendant nodes
};
// Start observing the target node
observer.observe(targetNode, config);
// Dynamically update the DOM
setTimeout(() => {
const newElement = document.createElement('p');
newElement.textContent = 'Hello, world!';
targetNode.appendChild(newElement); // Observer detects this change
}, 2000);
Use Cases:
- Monitoring dynamic content updates in single-page applications.
- Detecting attribute changes for user preference settings (e.g., dark mode toggles).
- Enabling real-time collaboration features (e.g., Google Docs-like updates).
- Building live notification systems for user interfaces.
- Tracking changes to dynamic forms or user-generated content.
3. Intersection Observers
Intersection observers monitor when elements enter or leave the viewport. This is particularly useful for lazy-loading images or triggering animations.
Code Example:
// Select the element to observe
const targetElement = document.querySelector('#box');
// Create an IntersectionObserver instance
const observer = new IntersectionObserver((entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
console.log('Element is in the viewport:', entry.target);
} else {
console.log('Element is out of the viewport:', entry.target);
}
});
});
// Start observing the target element
observer.observe(targetElement);
Use Cases:
- Lazy-loading images to improve page load performance.
- Implementing infinite scroll for content-heavy websites.
- Triggering CSS animations or JavaScript actions when elements appear on screen.
- Tracking ad visibility for impression measurement.
- Prioritizing above-the-fold content for SEO purposes.
4. Resize Observers
Resize observers track changes to the size of an element, making them ideal for building responsive UIs.
Code Example:
// Select the element to observe
const box = document.querySelector('#resizable');
// Create a ResizeObserver instance
const observer = new ResizeObserver((entries) => {
entries.forEach((entry) => {
const { width, height } = entry.contentRect;
console.log(`New size: ${width}px x ${height}px`);
});
});
// Start observing the element
observer.observe(box);
// Dynamically change the size
setTimeout(() => {
box.style.width = '400px';
box.style.height = '200px';
}, 2000);
Use Cases:
- Adapting components to dynamic size changes in responsive designs.
- Automatically resizing chart or canvas elements.
- Dynamically applying media queries based on element dimensions.
- Monitoring resizable panels in a split-view layout.
- Adjusting layouts for user customization features.
5. Object Property Observers
Object property changes can be observed using the Proxy
API, allowing you to intercept and react to property updates dynamically.
Code Example:
// Create an object to observe
const obj = { name: 'John', age: 30 };
// Use Proxy to intercept changes
const observedObj = new Proxy(obj, {
set(target, property, value) {
console.log(`Property ${property} changed from ${target[property]} to ${value}`);
target[property] = value; // Set the new value
return true;
},
});
// Change properties to trigger the observer
observedObj.name = 'Doe'; // Logs: Property name changed from John to Doe
observedObj.age = 31; // Logs: Property age changed from 30 to 31
Use Cases:
- Observing and validating changes to application state objects.
- Debugging property updates in complex state management systems.
- Restricting unauthorized updates to sensitive data.
- Creating reactive form models for data binding.
- Implementing dynamic validation logic based on property changes.
6. Observable Patterns (RxJS)
RxJS provides a robust implementation of the observer pattern, allowing you to create and manage data streams efficiently.
Code Example:
import { Observable } from 'rxjs';
// Create an observable
const observable = new Observable((subscriber) => {
subscriber.next('First value');
setTimeout(() => subscriber.next('Second value'), 1000);
setTimeout(() => subscriber.complete(), 2000); // End the stream
});
// Subscribe to the observable
observable.subscribe({
next: (value) => console.log('Received:', value),
complete: () => console.log('Stream complete'),
});
Use Cases:
- Managing asynchronous data streams like HTTP requests or WebSocket events.
- Handling real-time updates in dashboards or notifications.
- Implementing reactive programming patterns in front-end frameworks.
- Coordinating multiple asynchronous operations with ease.
- Building reusable utilities for event debouncing or throttling.
7. Performance Observers
Performance observers monitor performance-related events, such as resource loading or long tasks, to optimize application performance.
Code Example:
// Create a PerformanceObserver instance
const observer = new PerformanceObserver((list) => {
const entries = list.getEntries();
entries.forEach((entry) => {
console.log('Performance entry:', entry);
});
});
// Start observing resource timing entries
observer.observe({ entryTypes: ['resource'] });
Use Cases:
- Debugging and analyzing web performance issues.
- Measuring resource load times for optimization.
- Identifying long tasks that block the main thread.
- Monitoring user-experience metrics like Time to Interactive (TTI).
- Analyzing performance impact of third-party scripts.
8. Custom Observers
Custom observers can be built using the observer design pattern for scenarios not covered by built-in APIs.
Code Example:
class Observable {
constructor() {
this.observers = [];
}
// Subscribe to the observable
subscribe(observer) {
this.observers.push(observer);
}
// Notify all observers
notify(data) {
this.observers.forEach((observer) => observer(data));
}
}
// Create an observable instance
const observable = new Observable();
// Subscribe to notifications
observable.subscribe((data) => console.log('Observer 1:', data));
observable.subscribe((data) => console.log('Observer 2:', data));
// Notify observers
observable.notify('Hello, Observers!');
Use Cases:
- Creating custom event systems for modular applications.
- Implementing reactive architectures for state management.
- Simplifying pub-sub models for decoupled components.
- Supporting application-specific notification mechanisms.
- Building dynamic workflows for user-driven actions.
Conclusion
Observers are versatile tools that form the backbone of many modern JavaScript applications. From handling user interactions to monitoring complex data streams, they enable developers to build responsive, dynamic, and user-friendly interfaces. By understanding and leveraging these observer types, you can take your JavaScript development to the next level.
Whether youโre building a small project or a large-scale application, these observer patterns and APIs will undoubtedly come in handy. Experiment with these examples to solidify your understanding, and donโt hesitate to incorporate them into your projects!
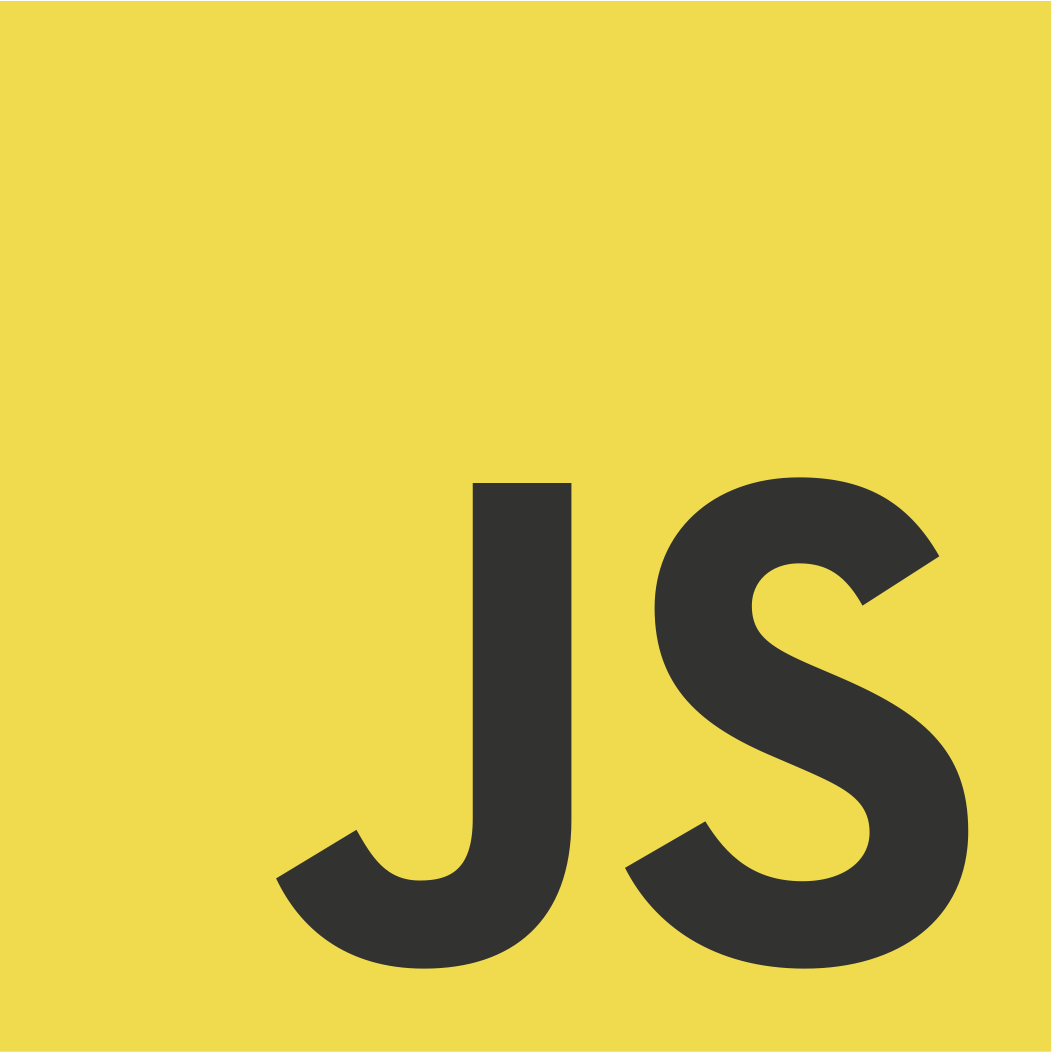
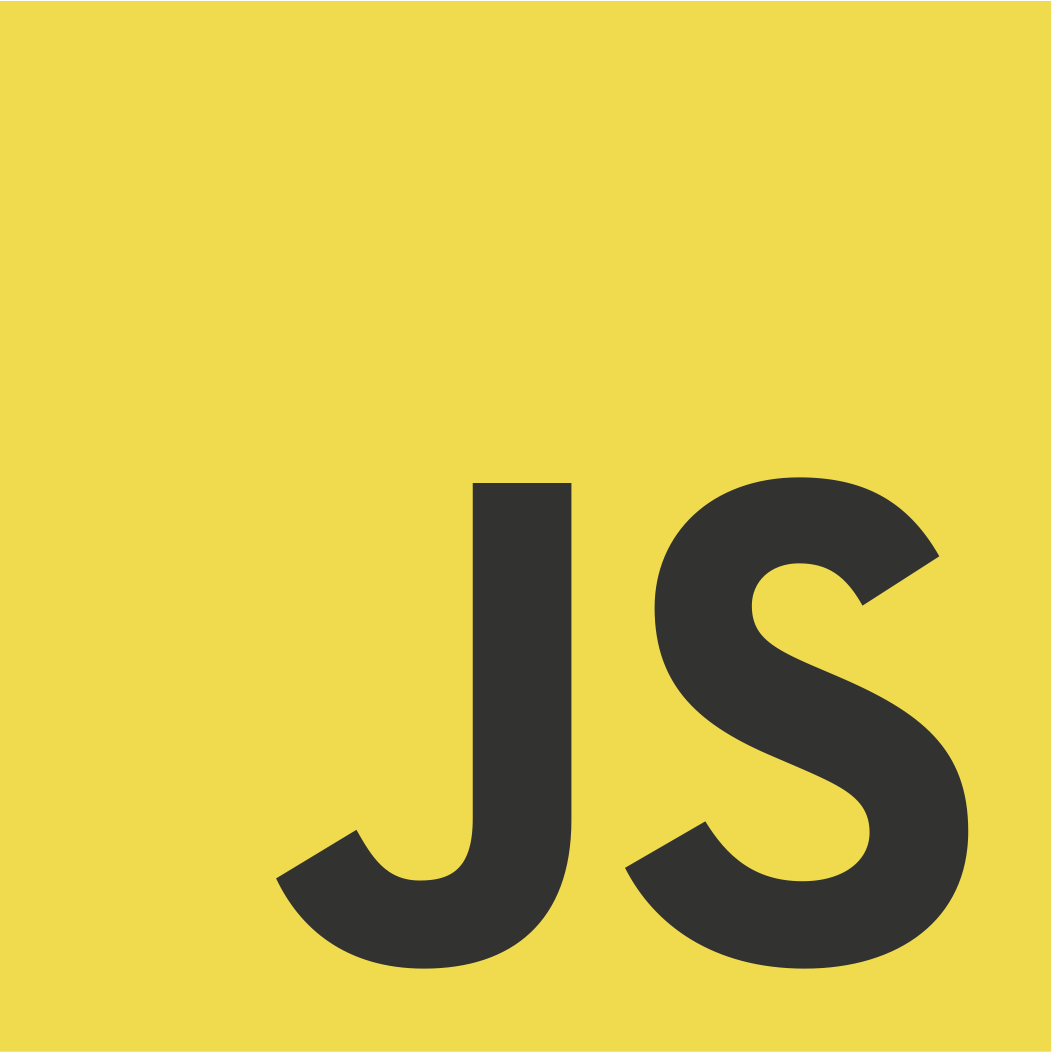
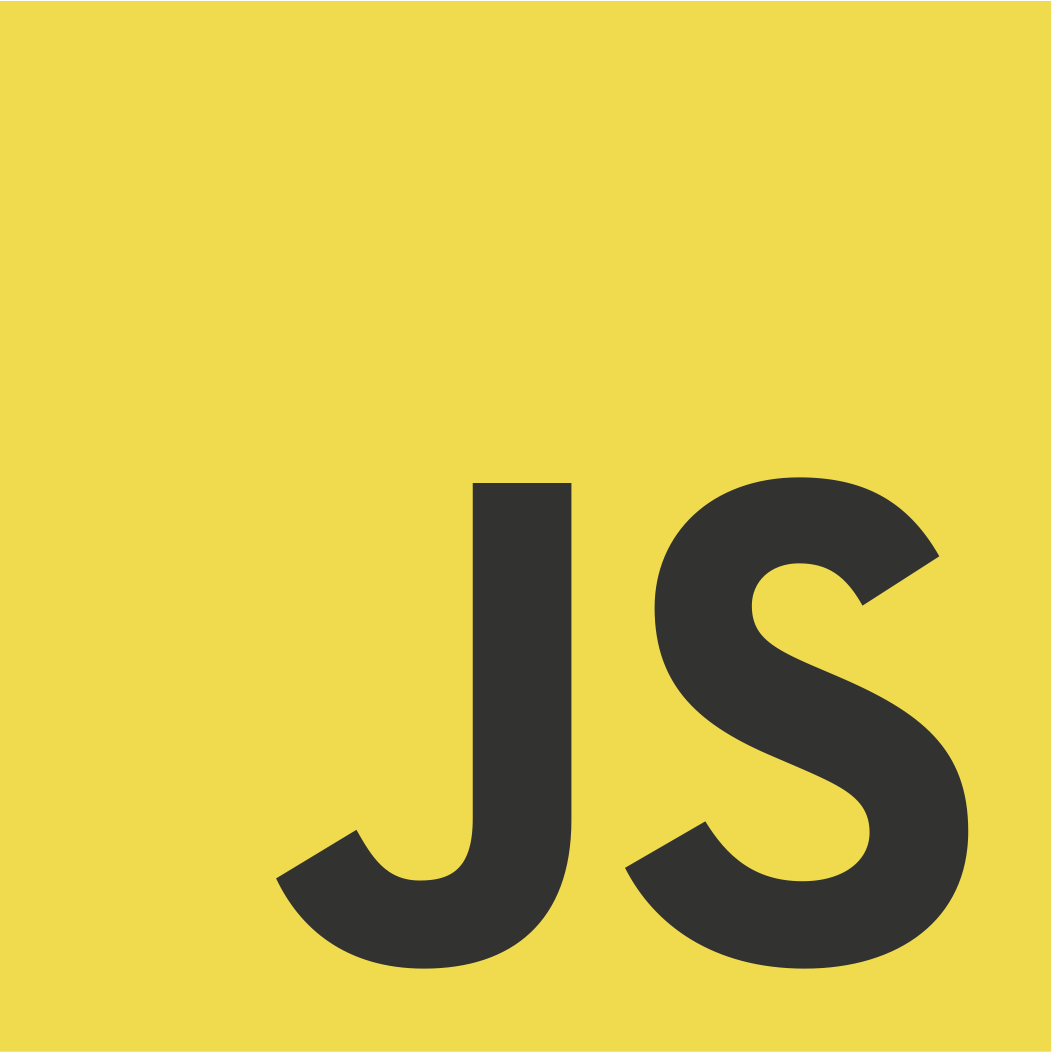
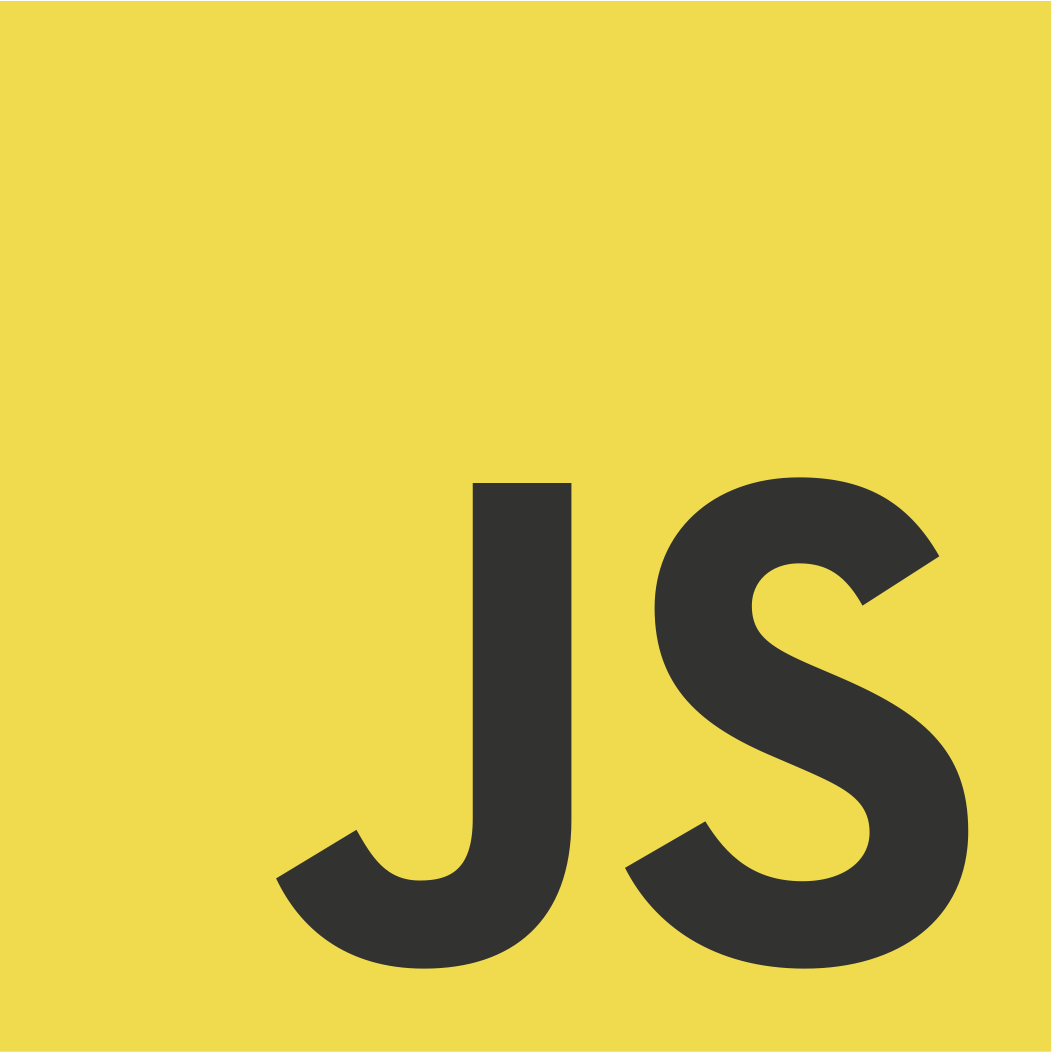
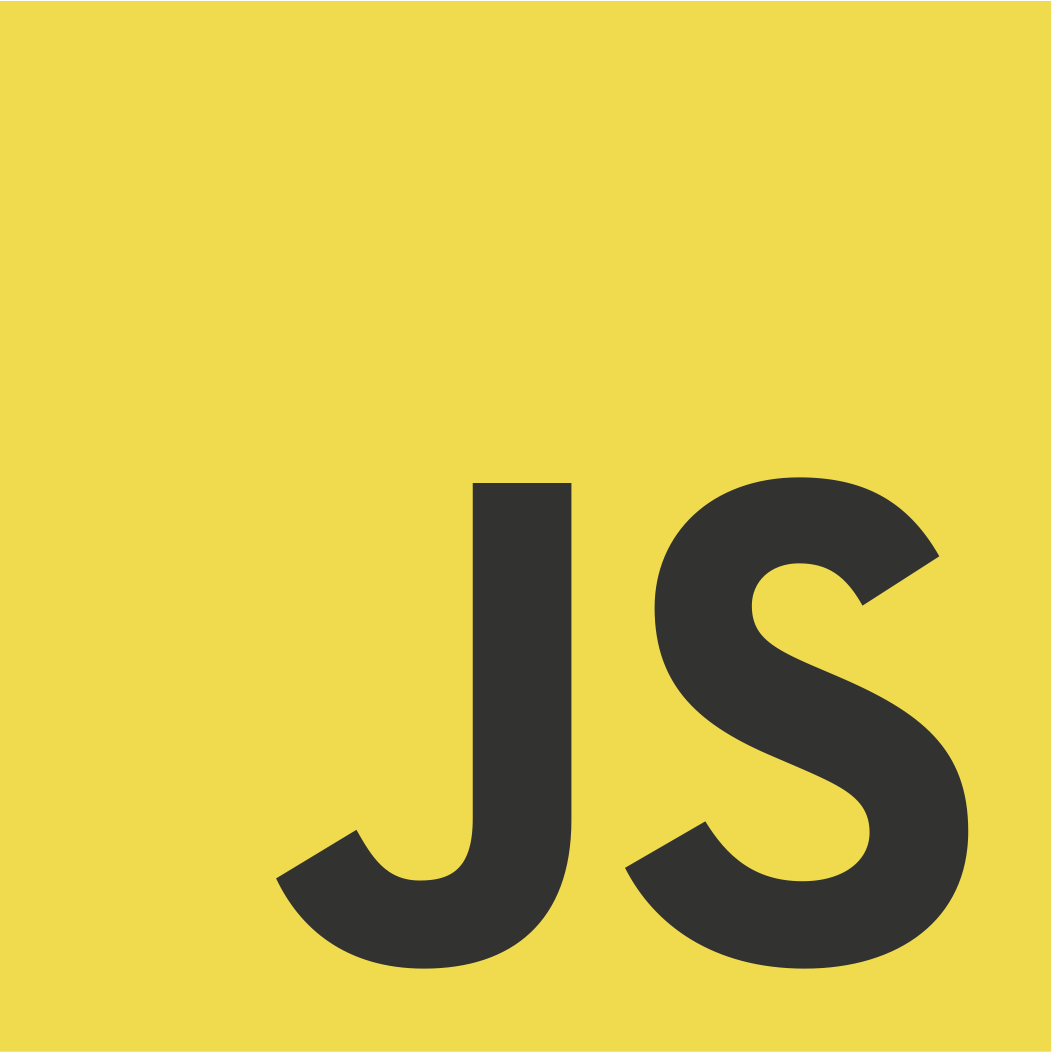
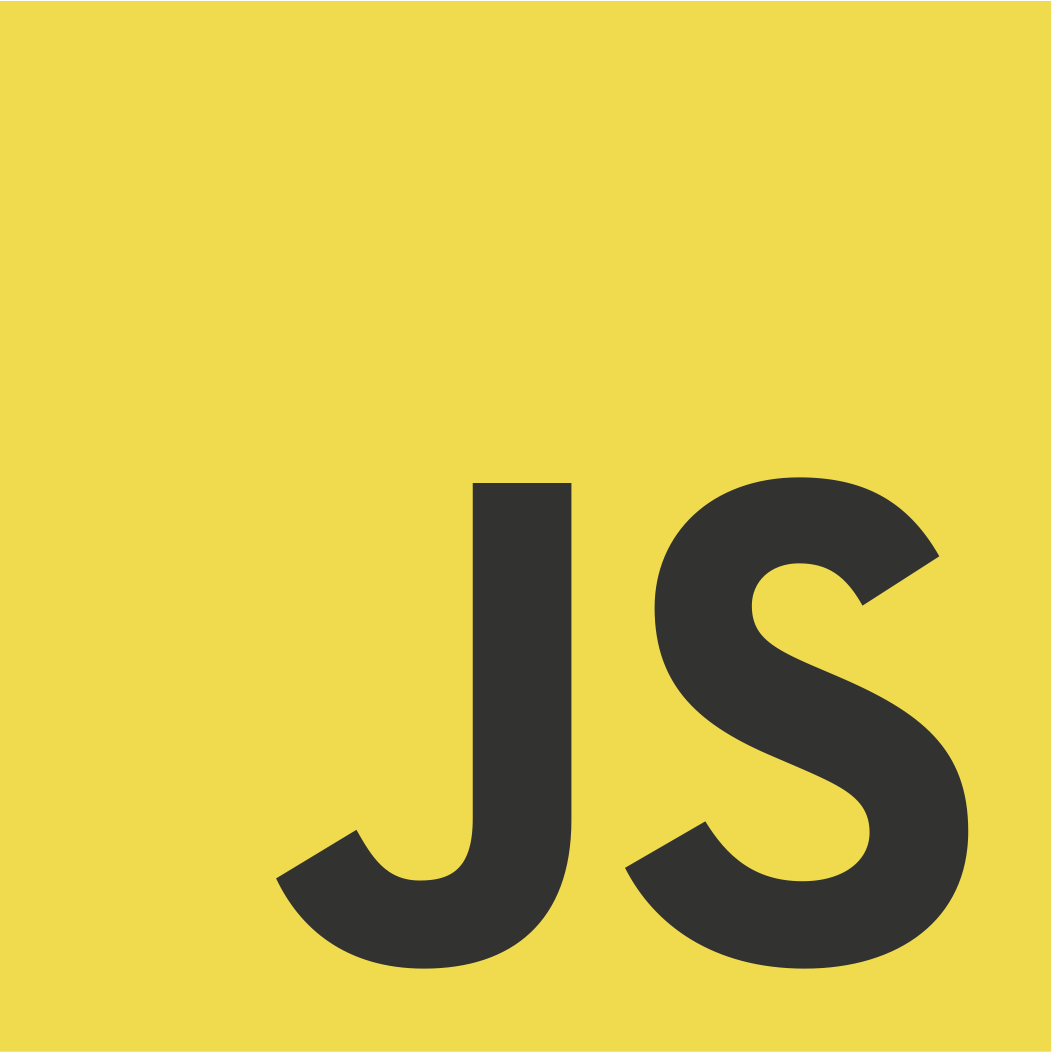
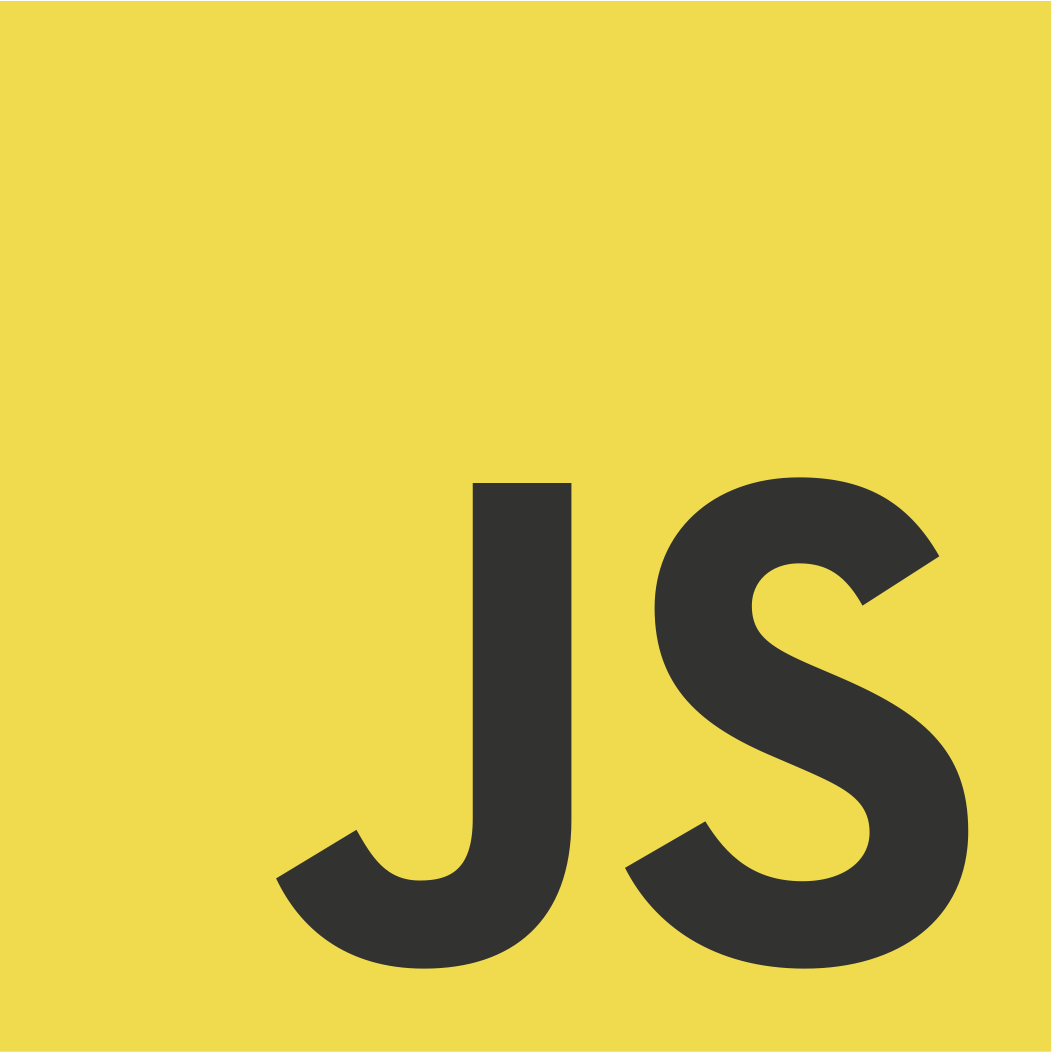
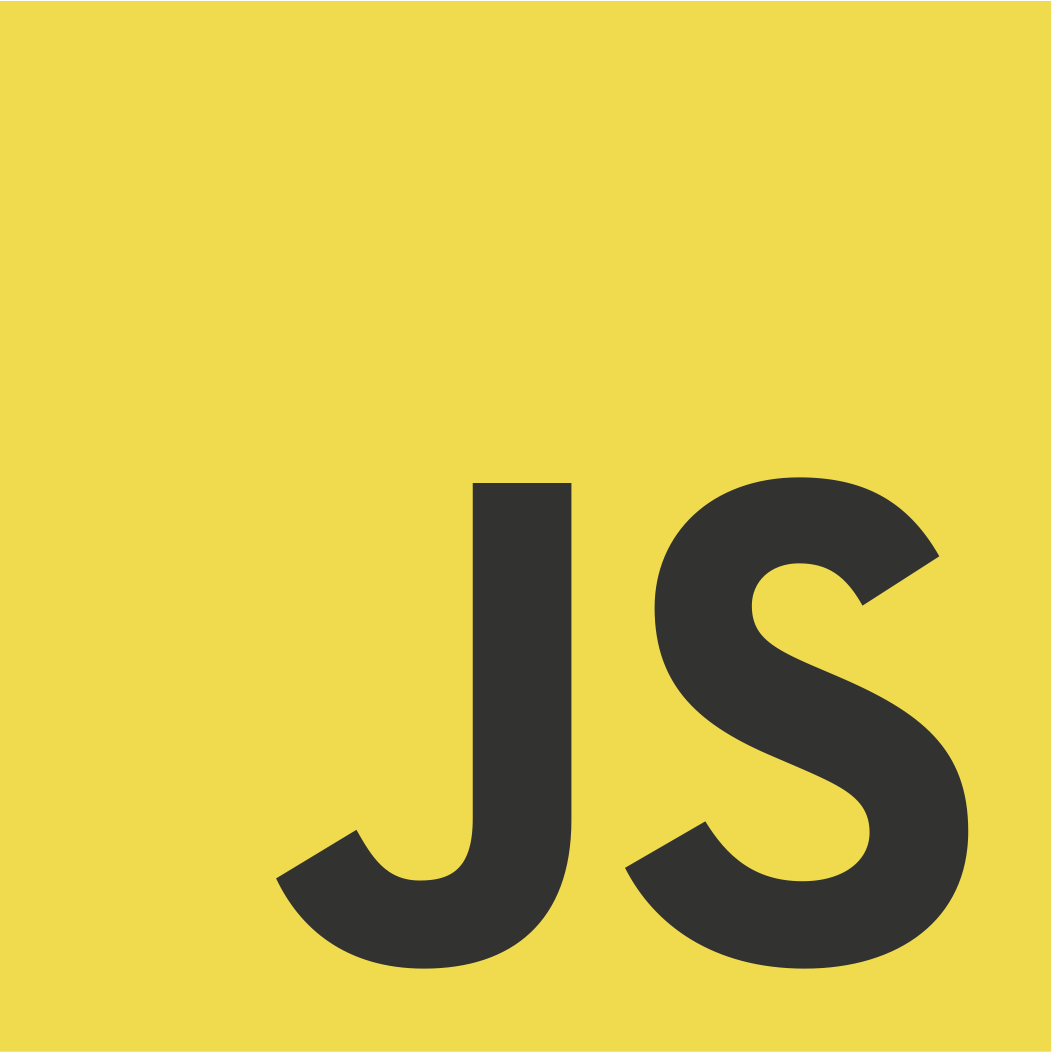
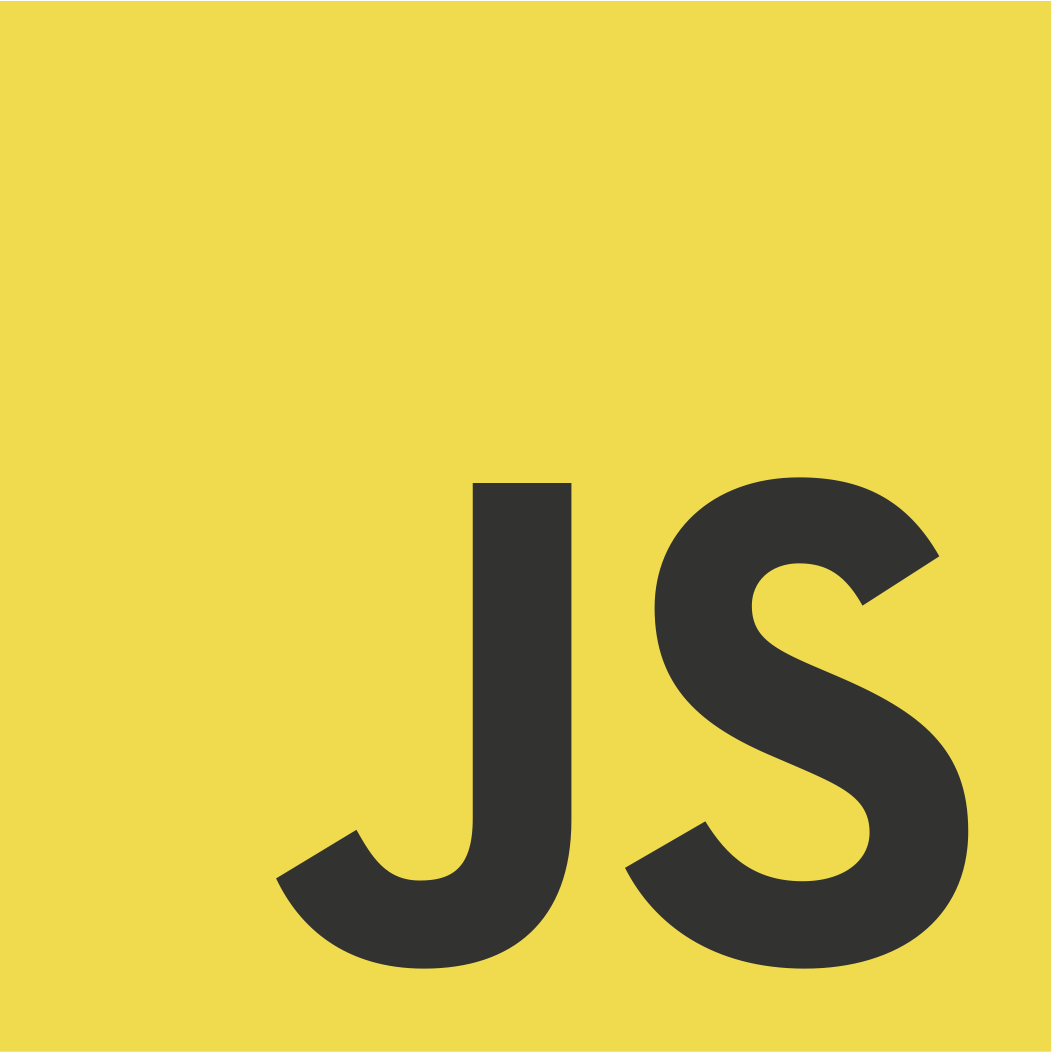
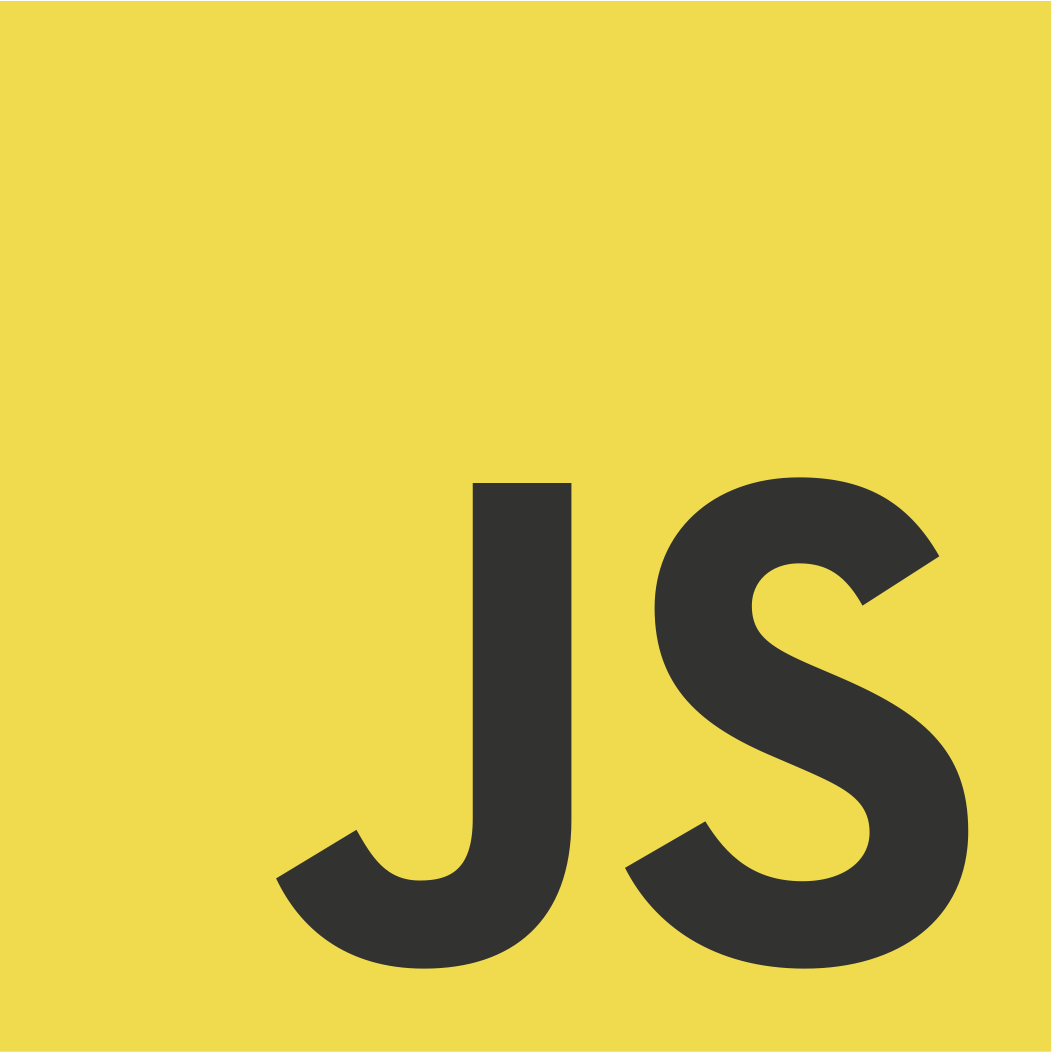
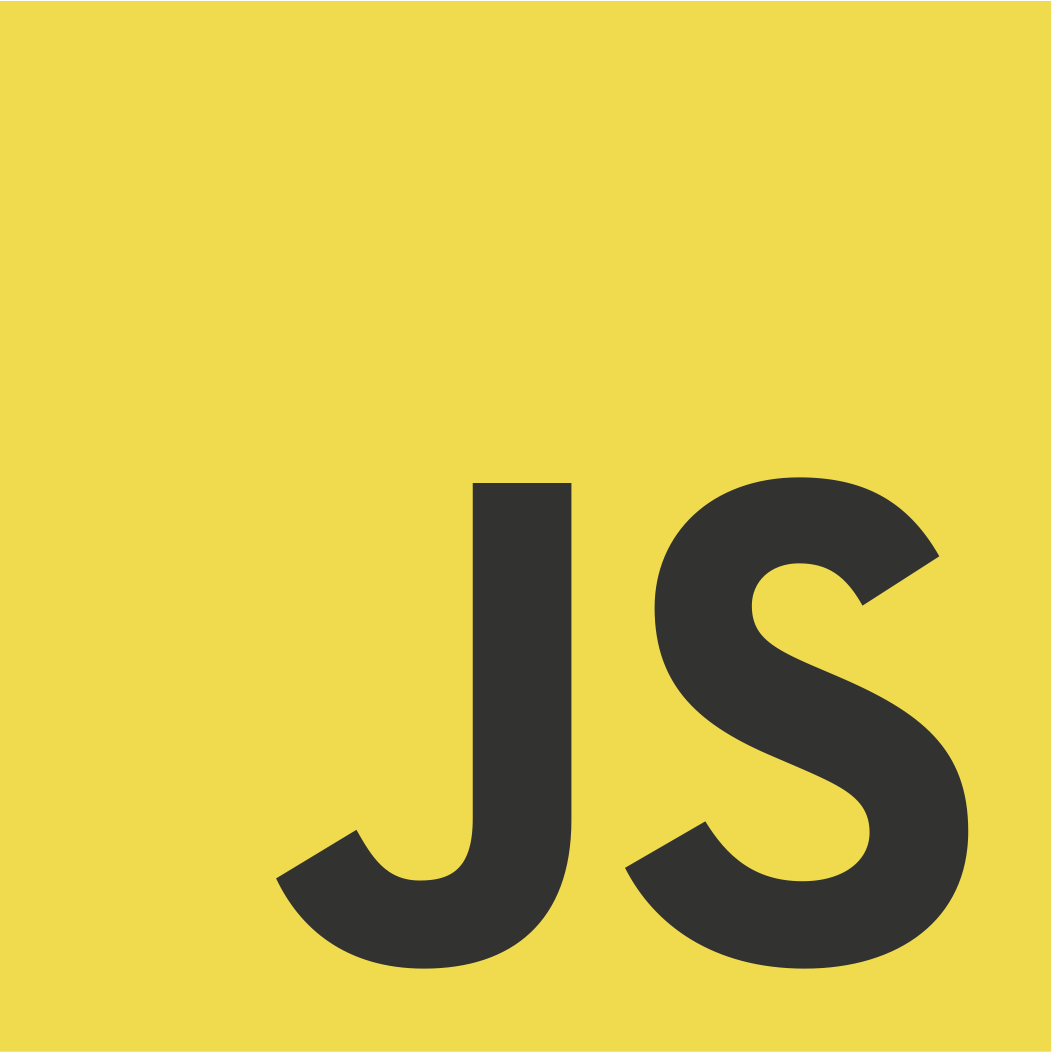
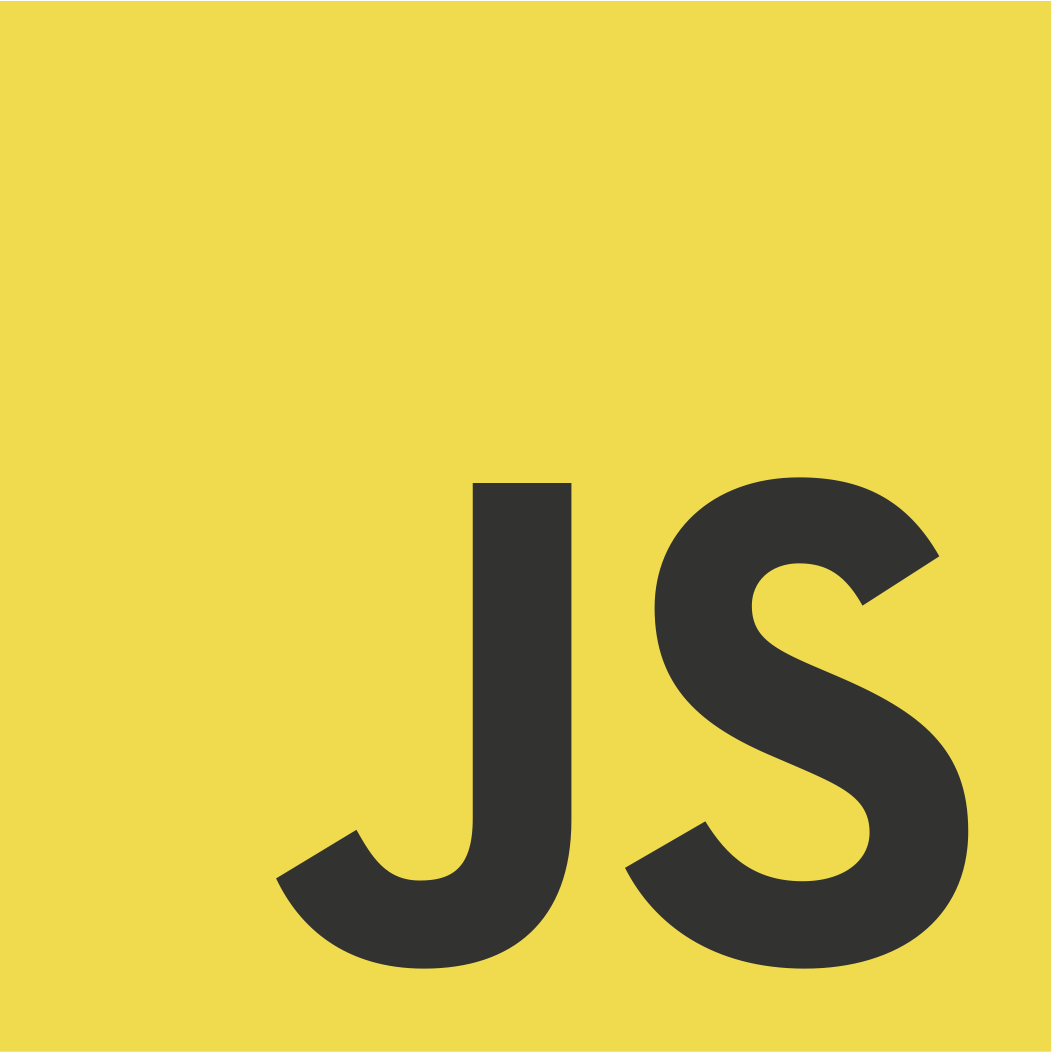
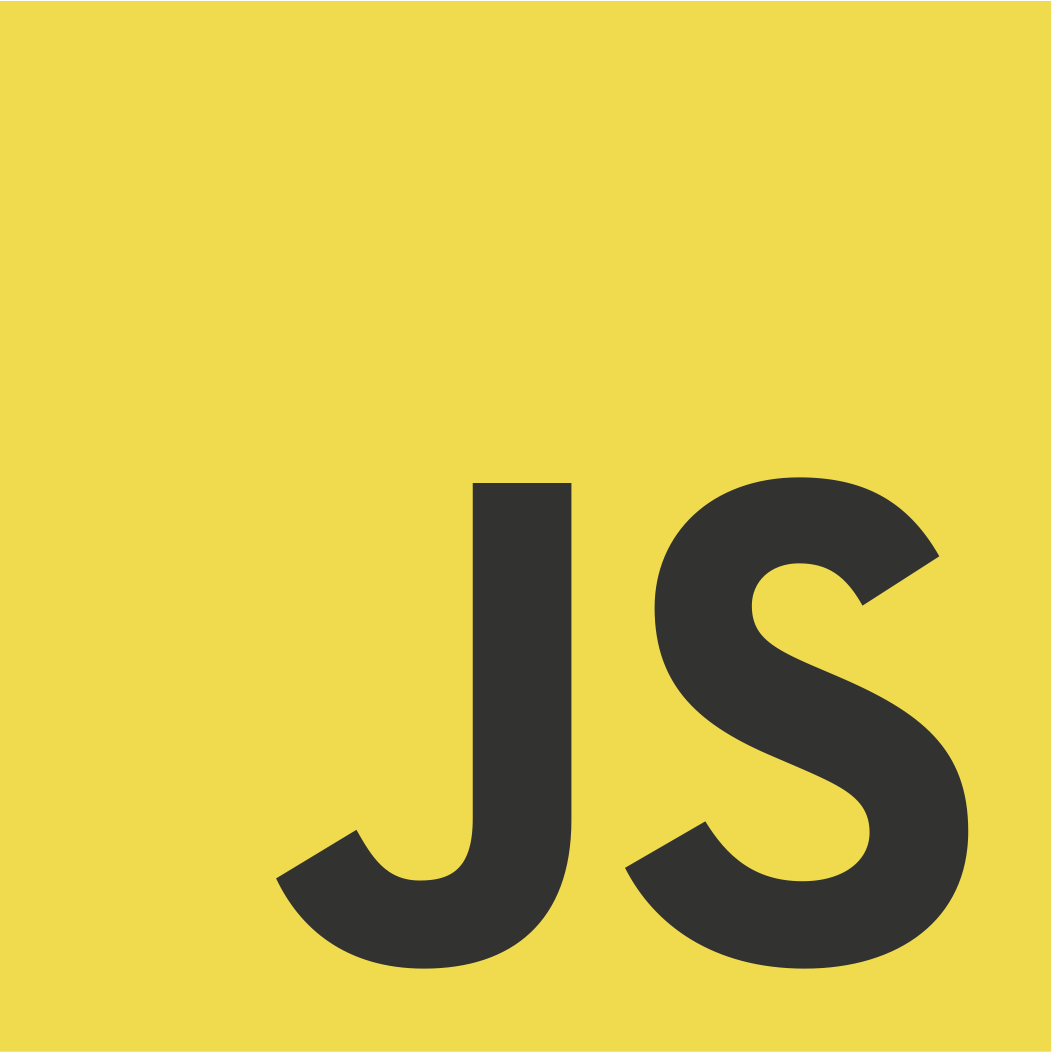
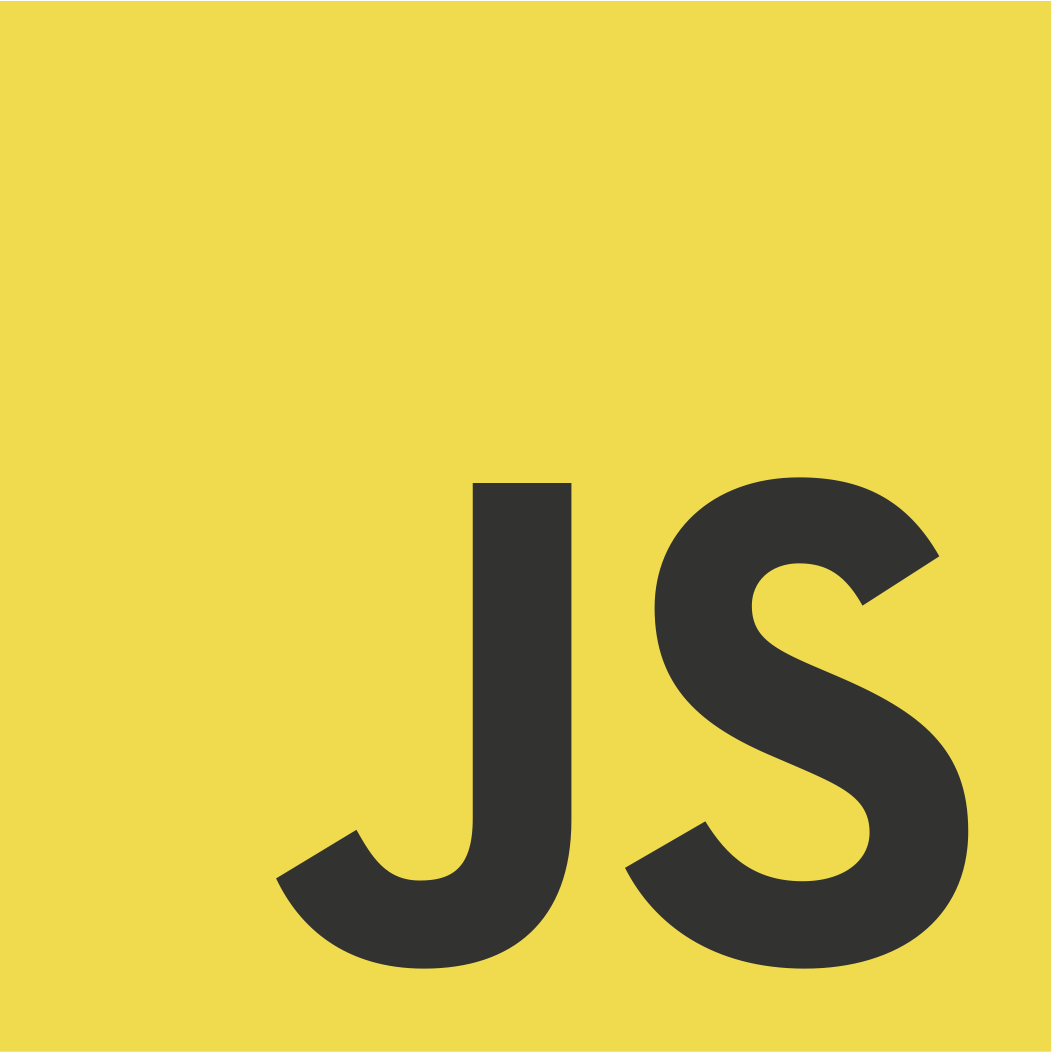
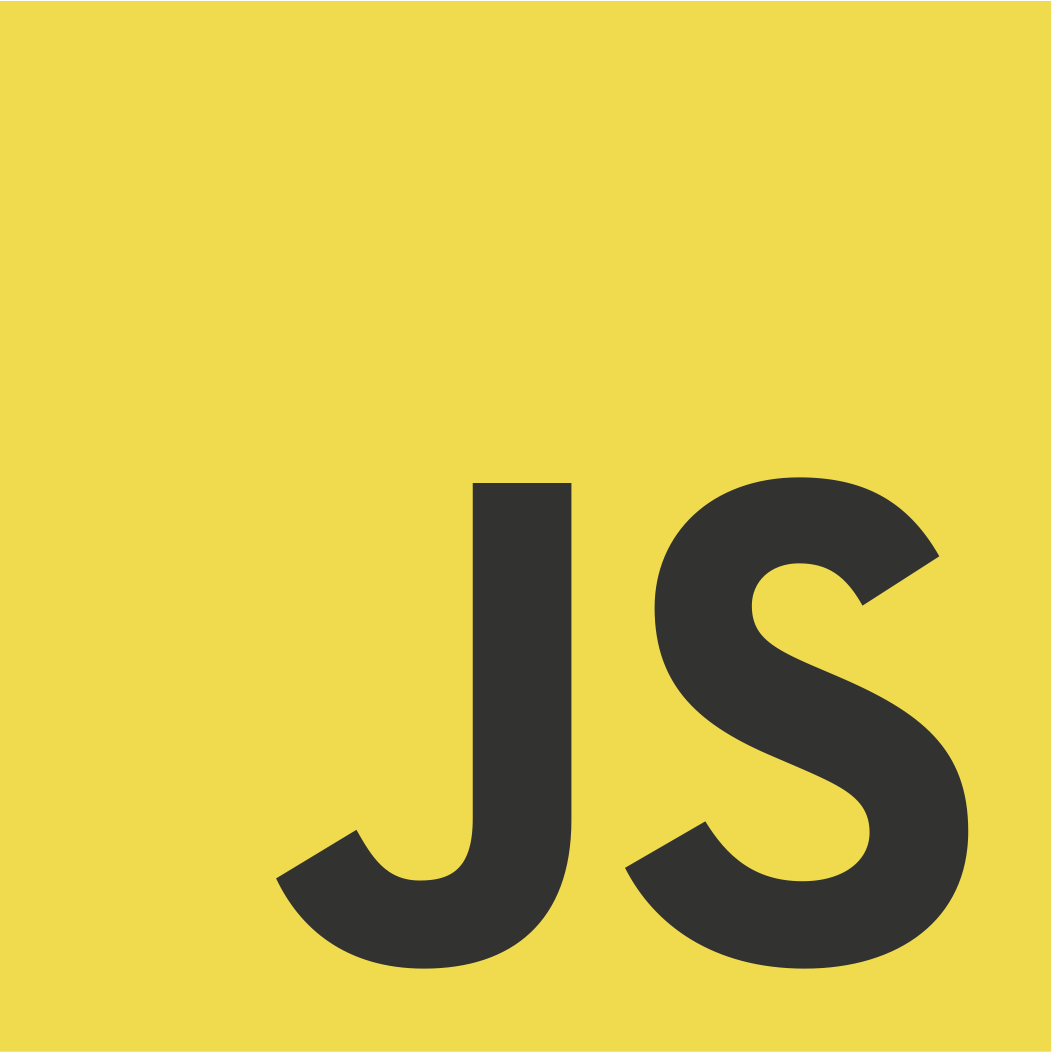
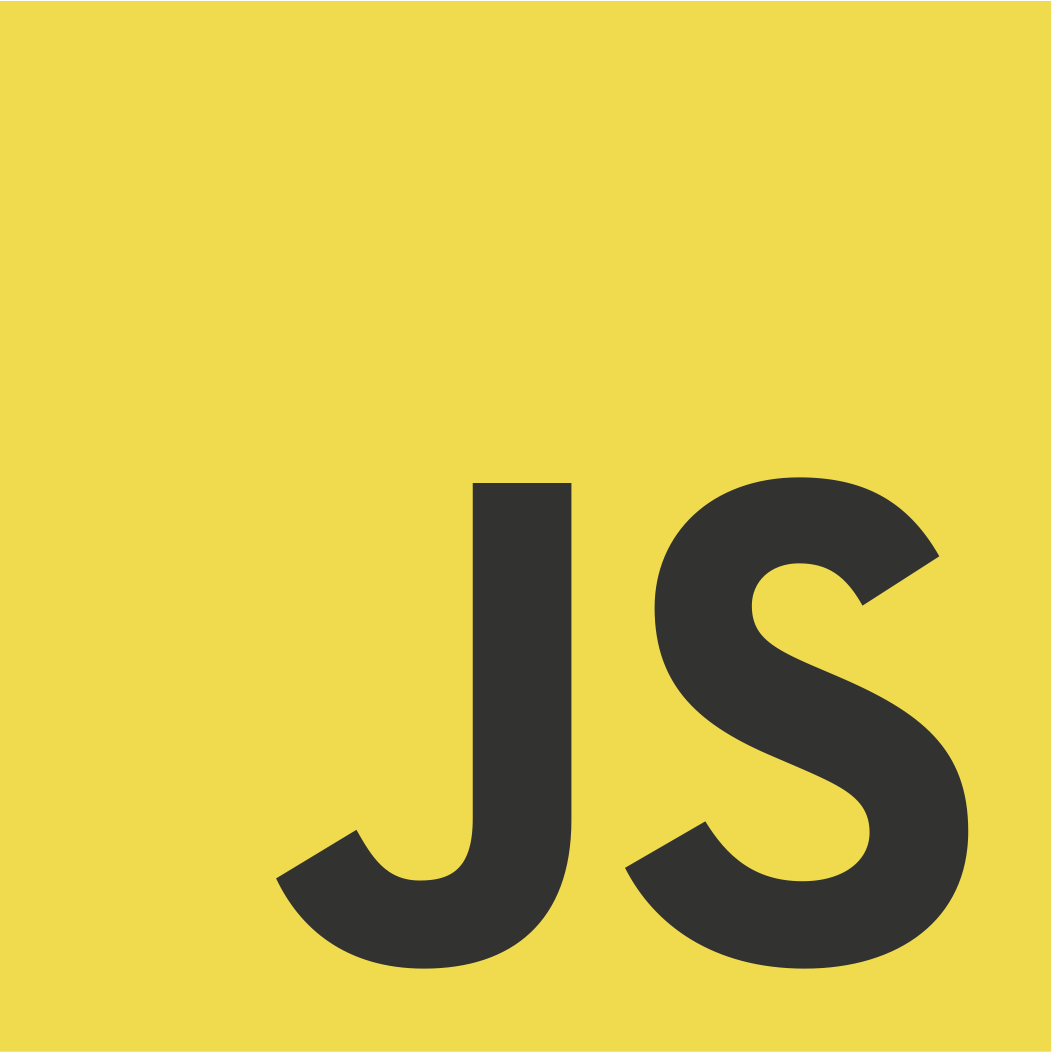
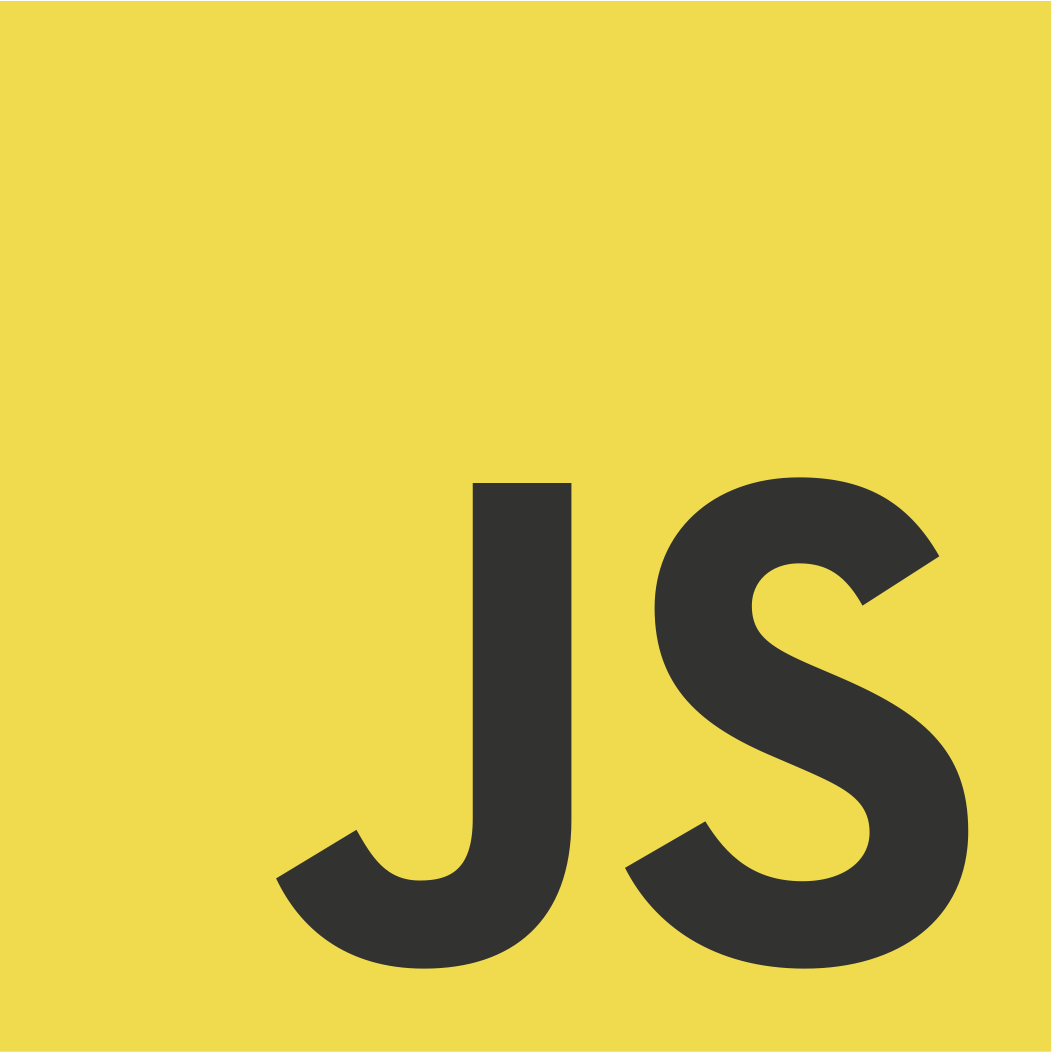
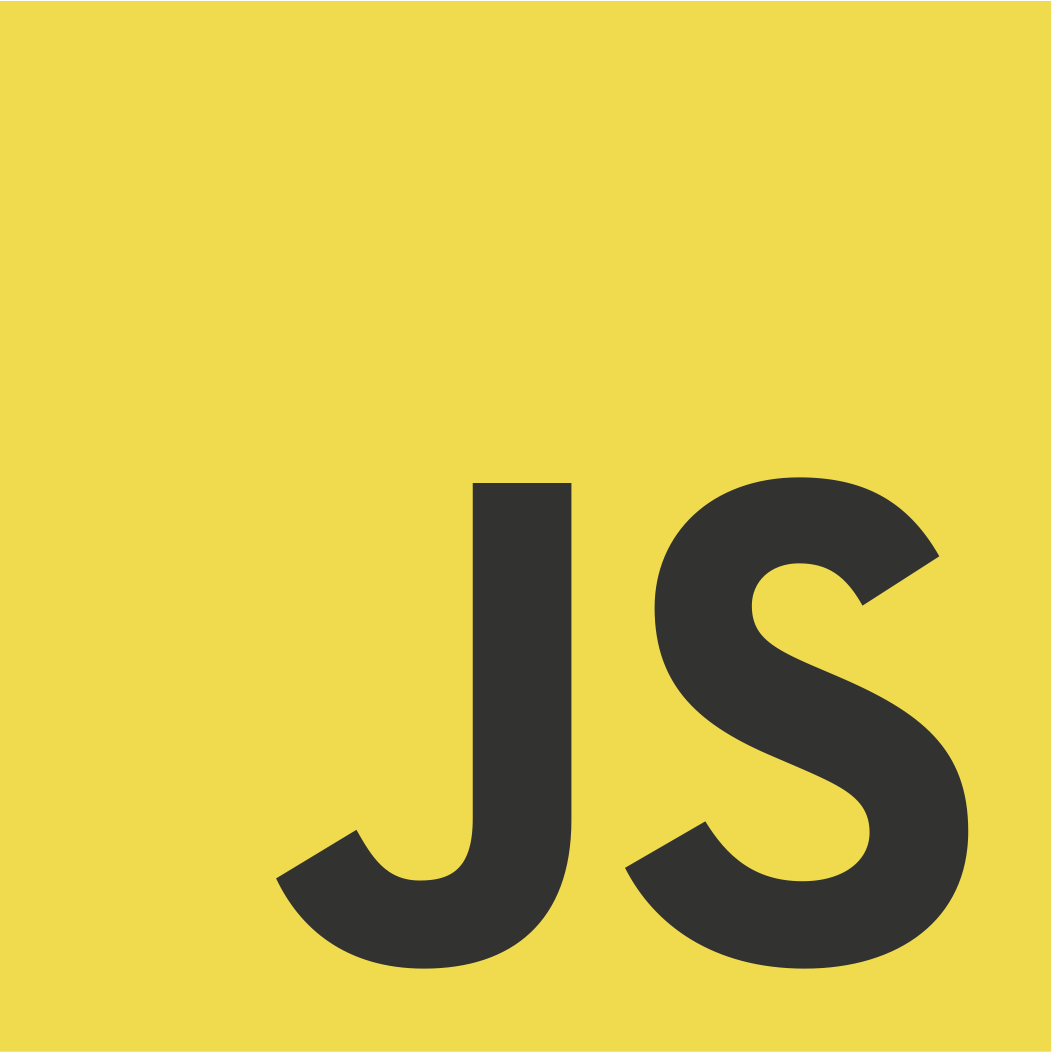
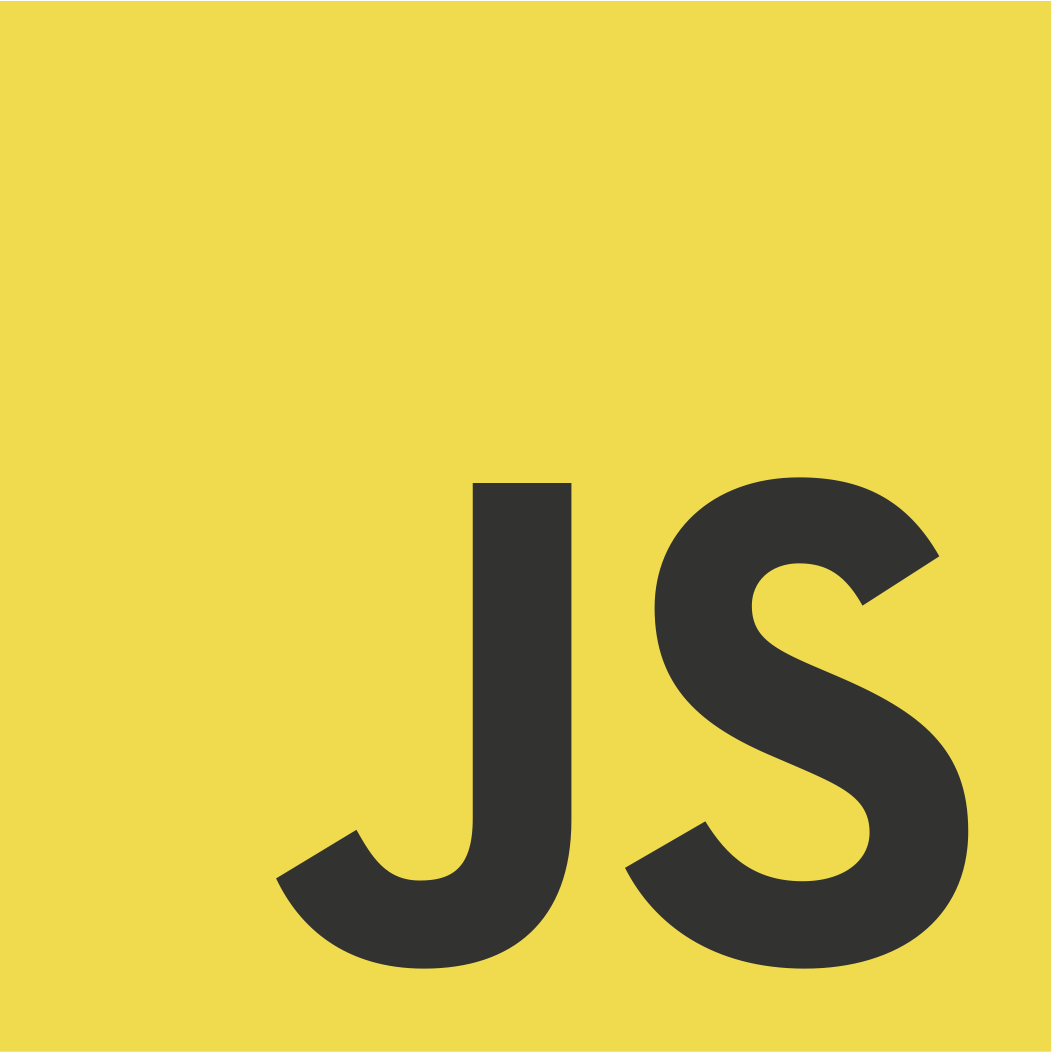
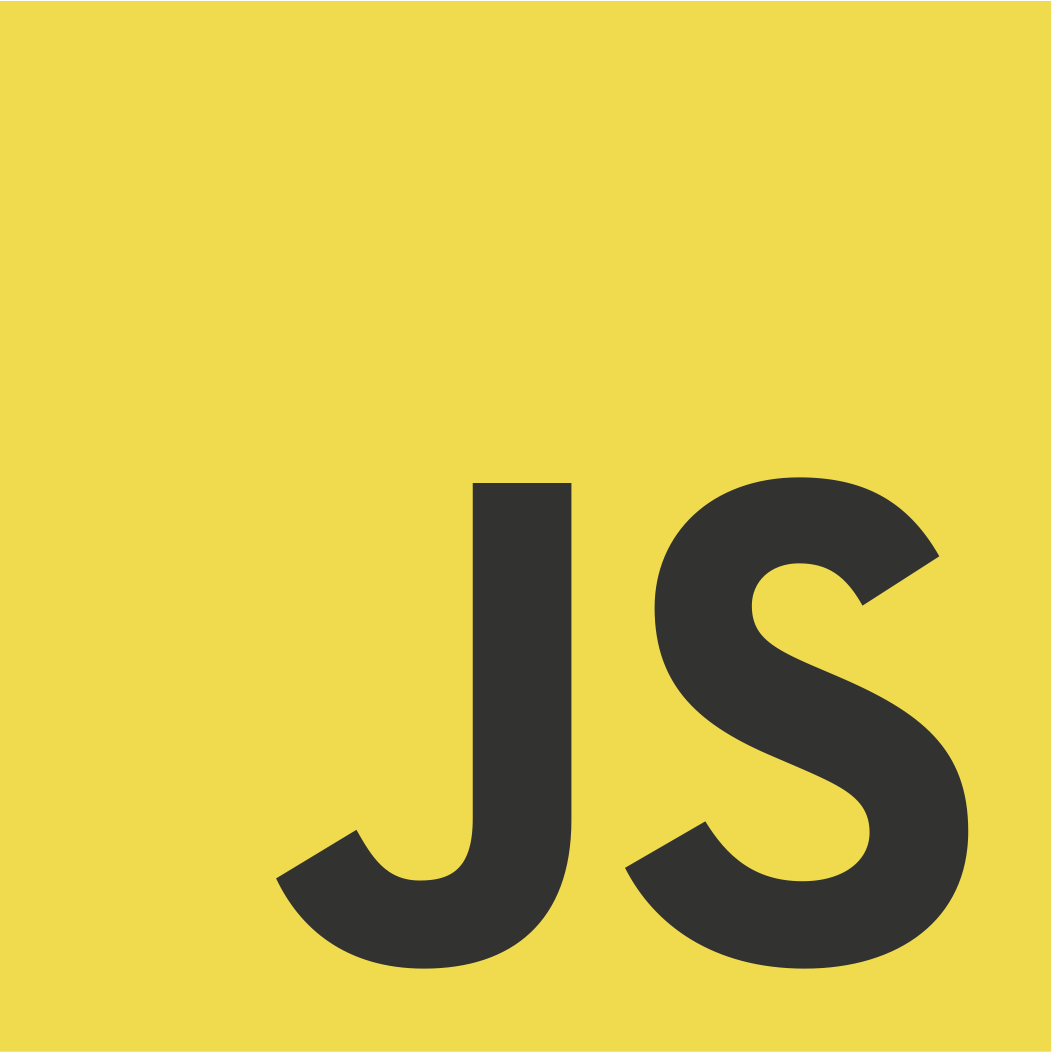
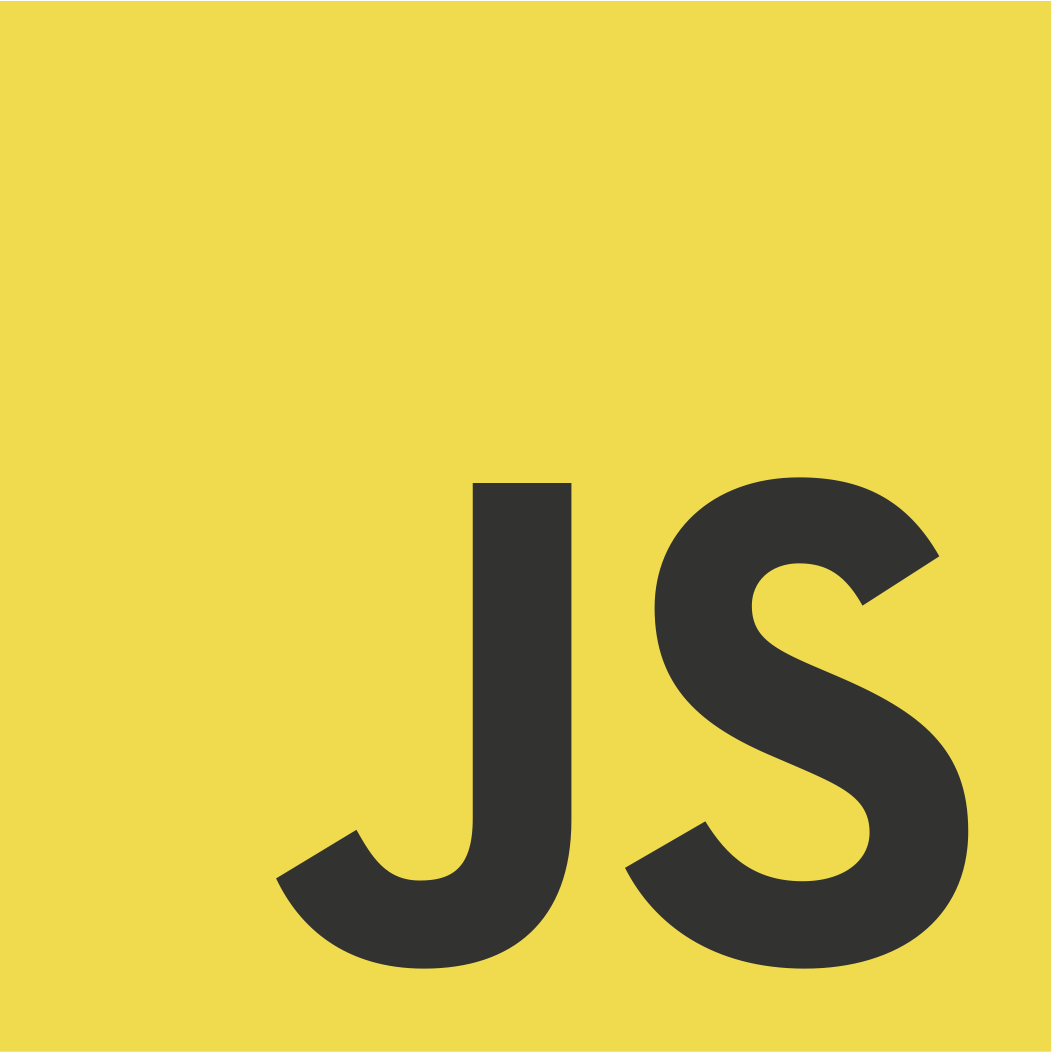
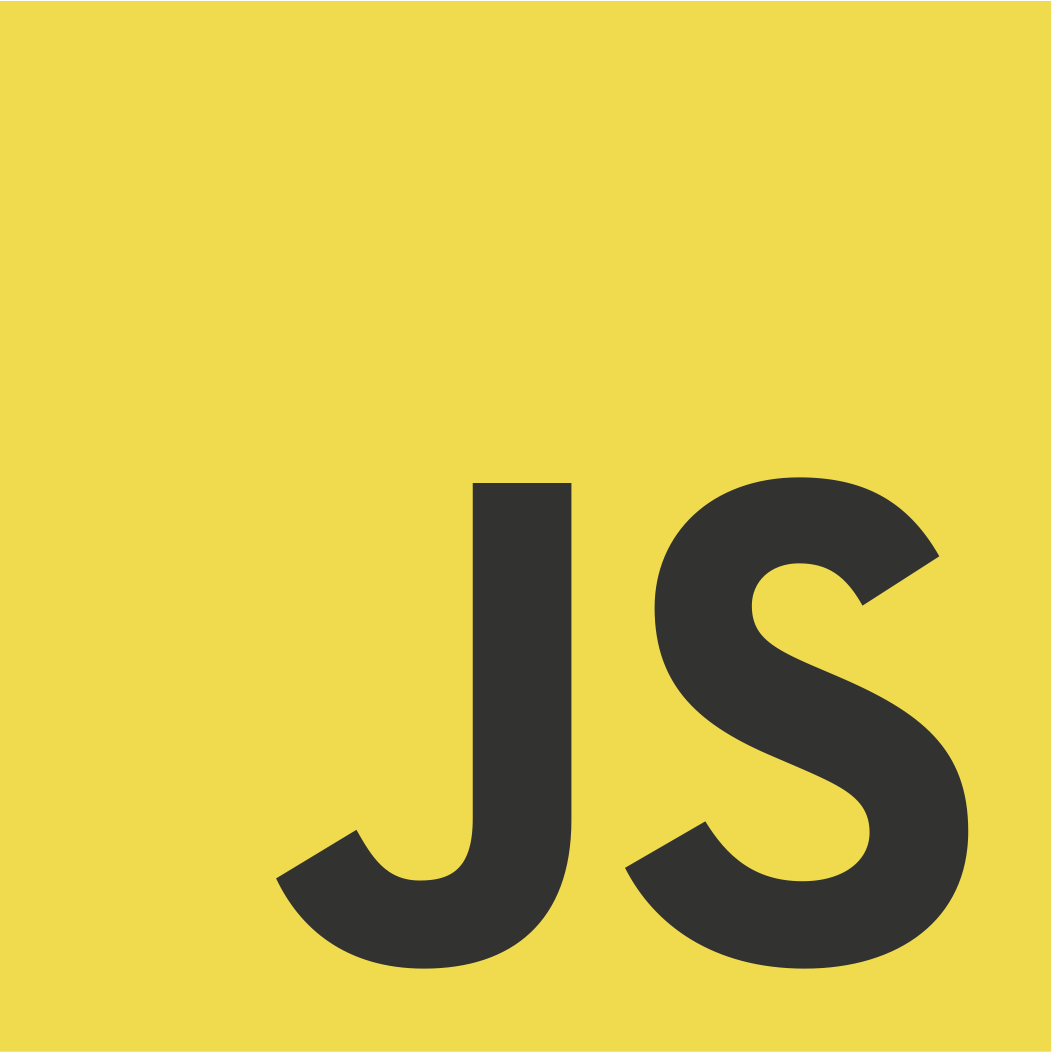
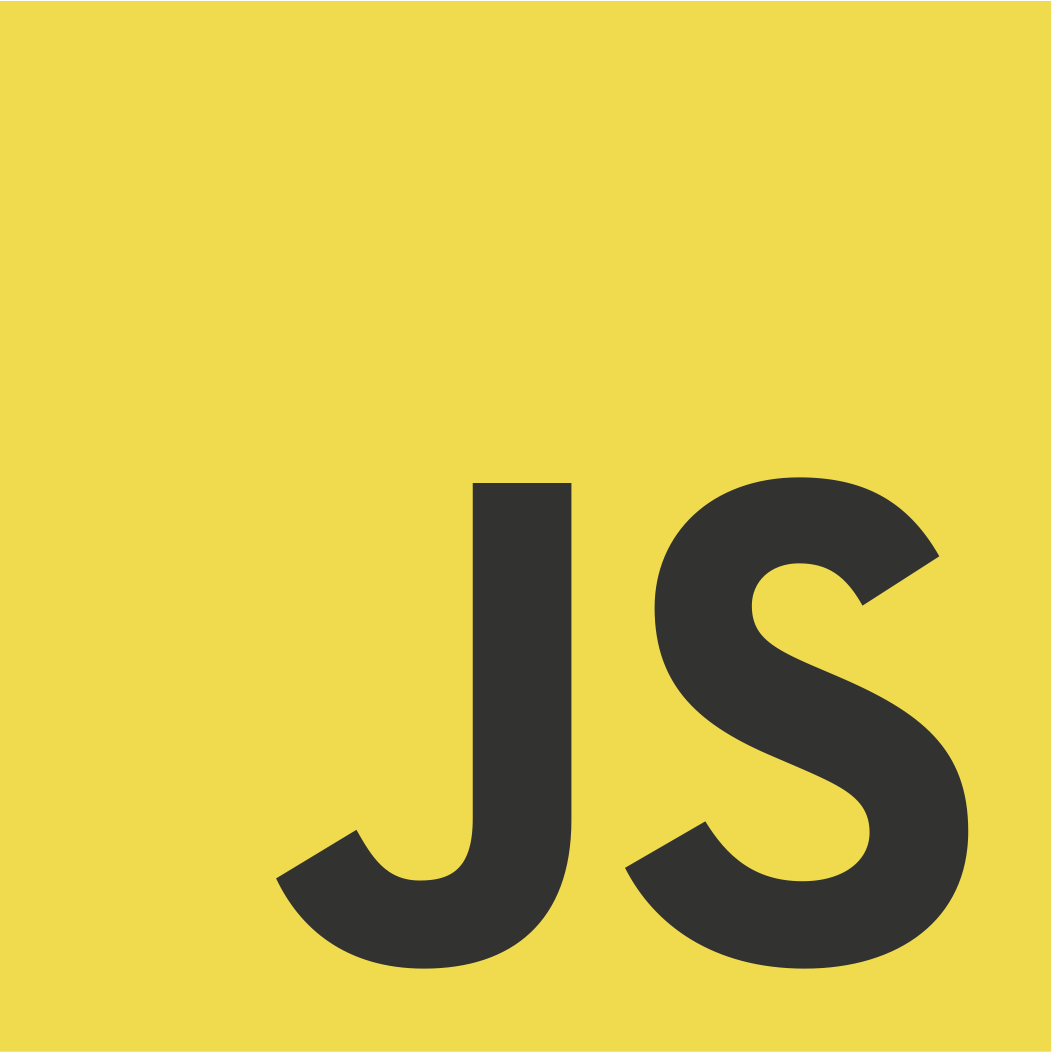
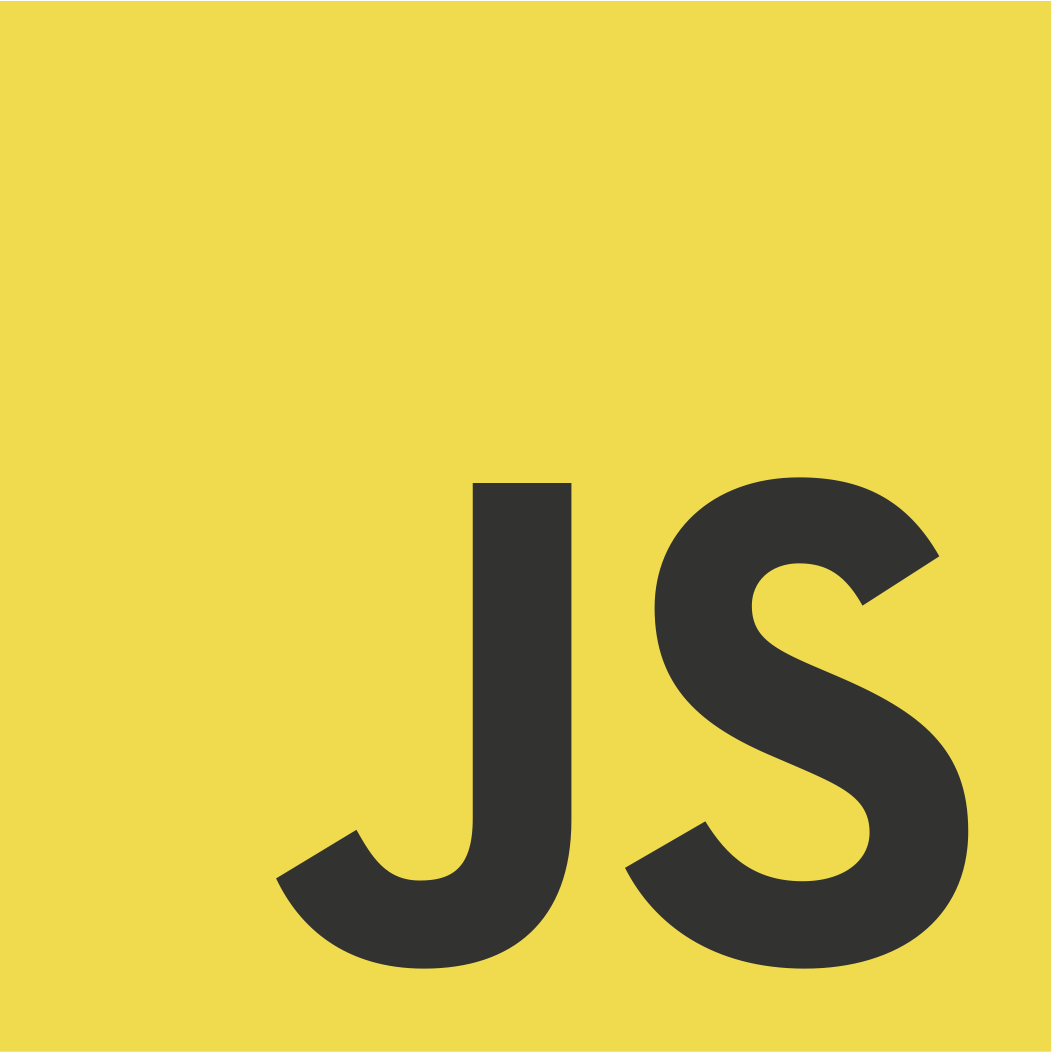
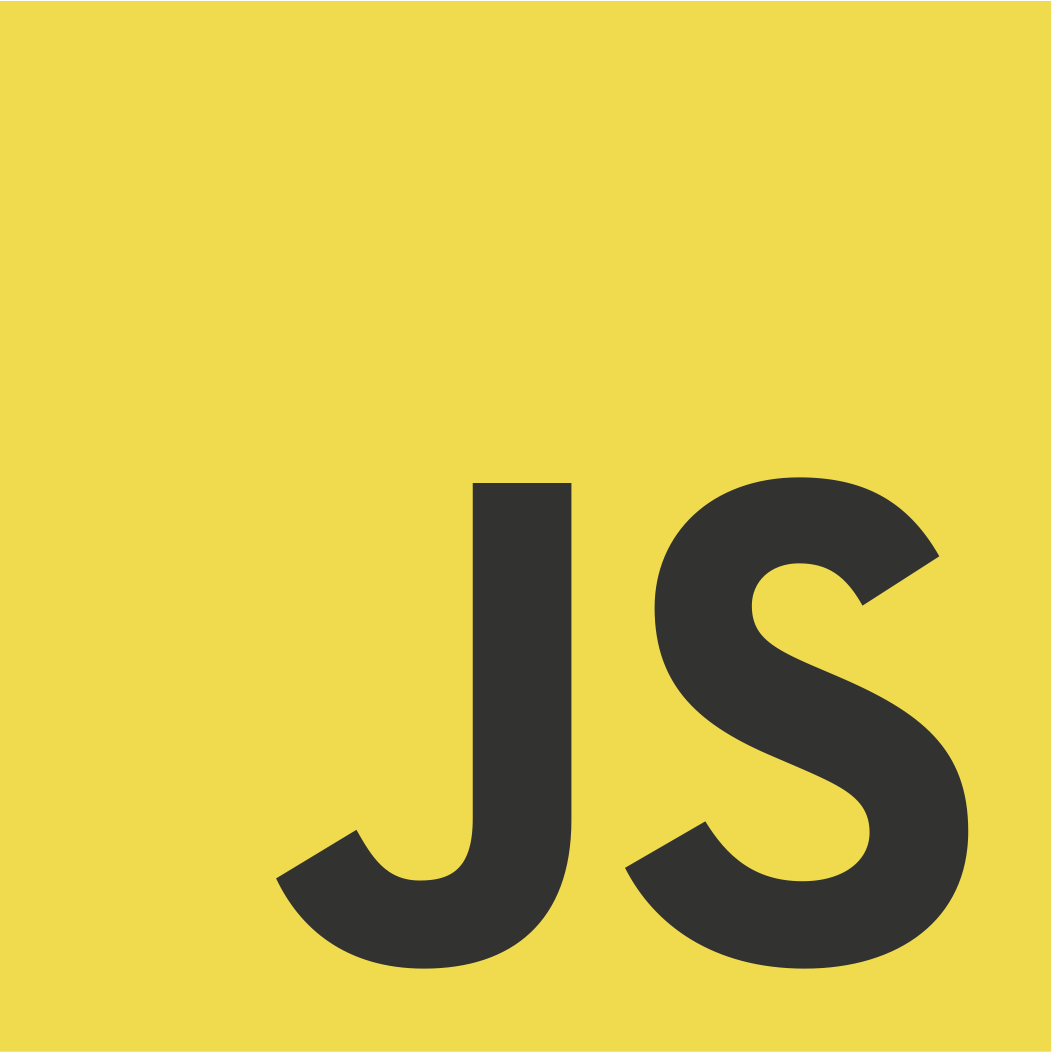
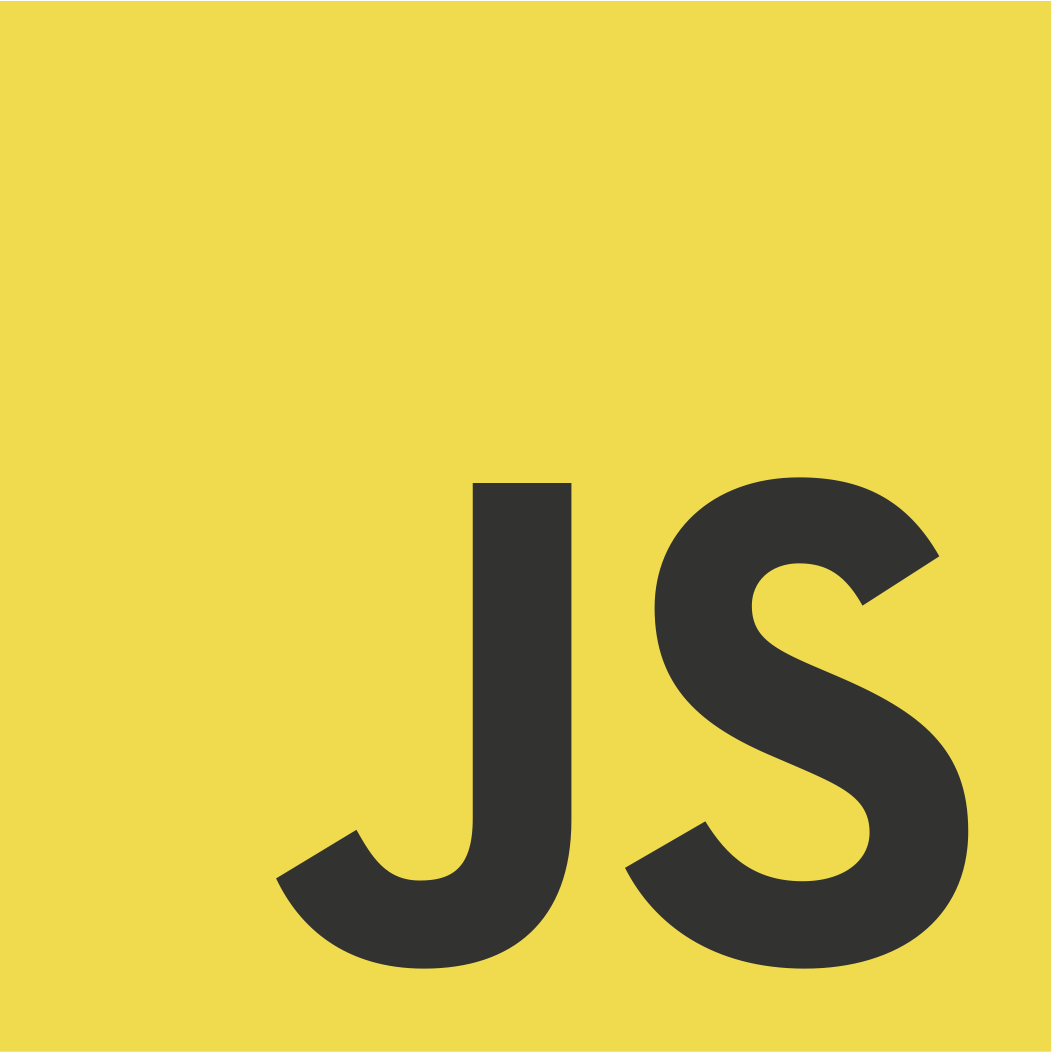
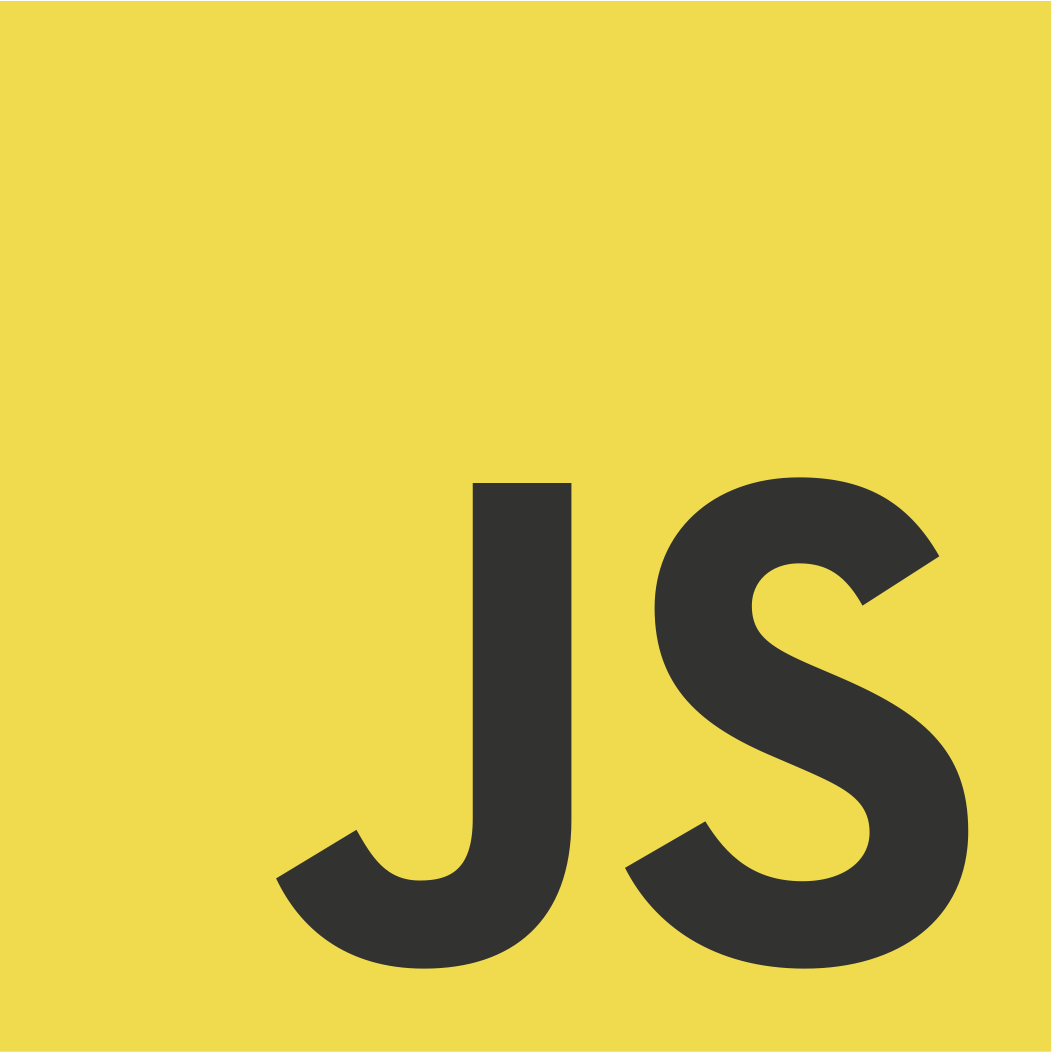
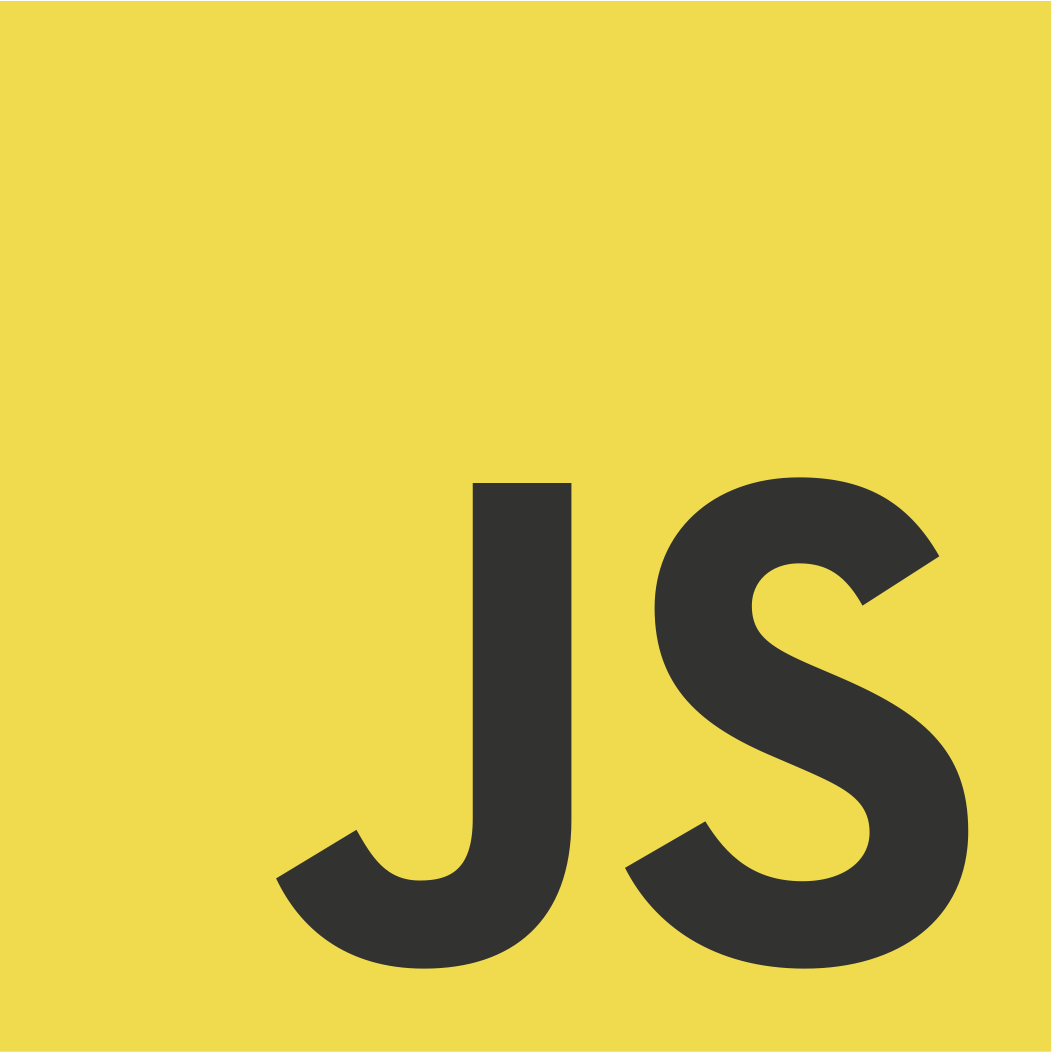
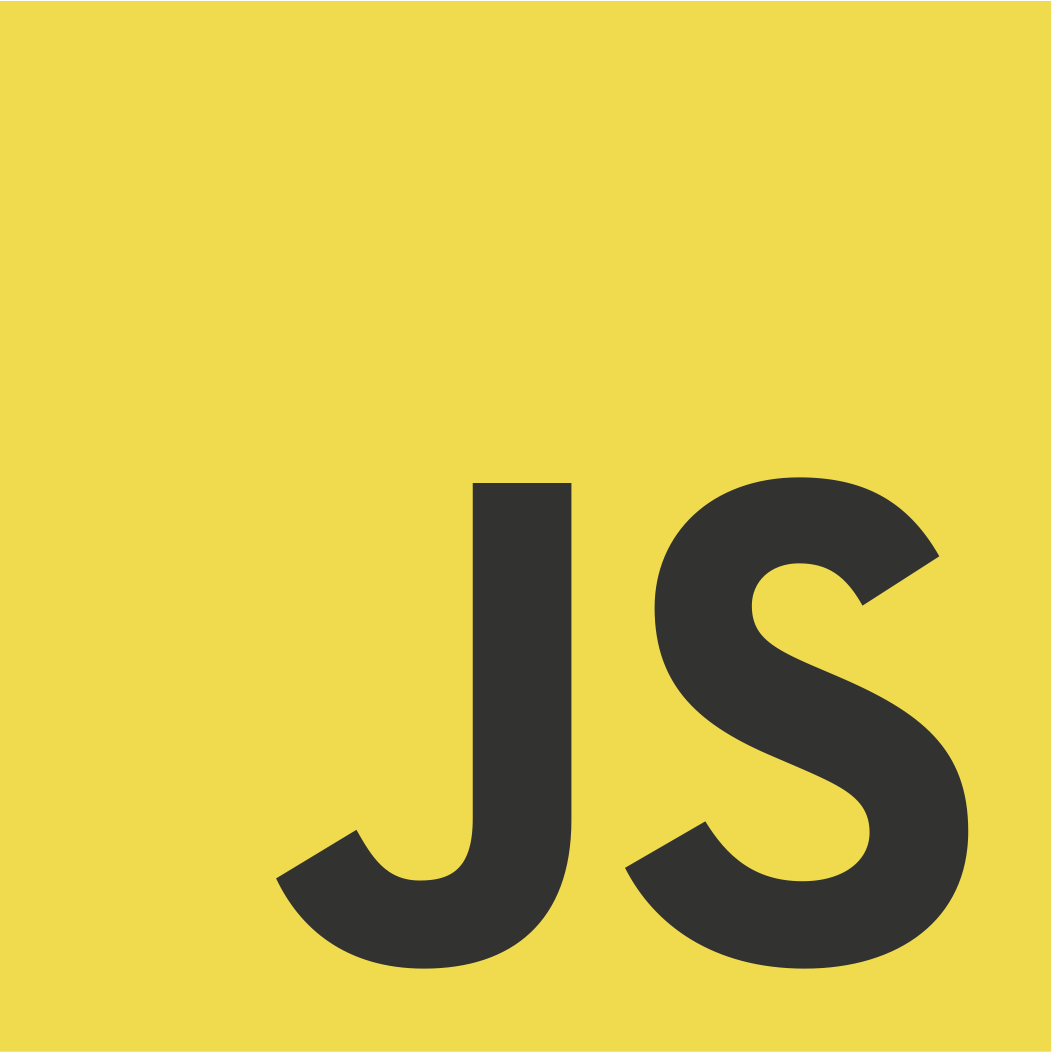
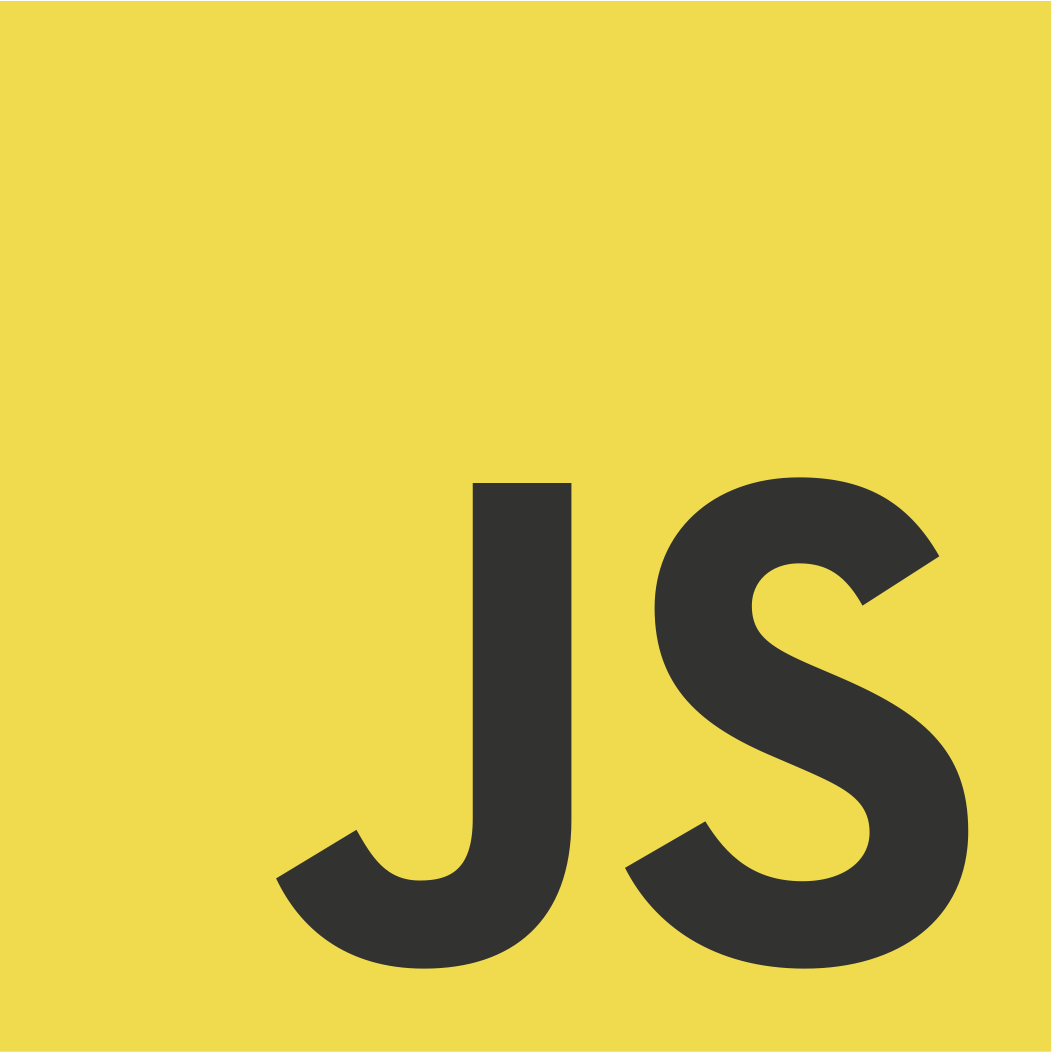
Featured ones: