
dev-resources.site
for different kinds of informations.
A Guide to Planning Your API: Code-First VS Design-First Approach
Picture yourself as an architect standing before an empty plot of land. You wouldn't start laying bricks without a blueprint, would you? The same principle applies to API development. I used to use the code-first approach which involves writing code first and then documenting it later until I learned the design-first approach. The design-first approach involves creating a detailed API definition before writing any code.
Your Journey Through This Guide
Before we dive in, let's map out where we're heading. Think of this as your API planning roadmap:
- Understanding API planning fundamentals
- Exploring two different approaches
- Making an informed choice
- Creating your API plan
What You'll Learn:
- What API Planning Involves
- The Code-First Approach
- The Design-First Approach
- Comparing Code-First and Design-First
- How to Choose the Right Approach
- Practical Steps to Plan Your API
What API Planning Involves
The Foundation of Great APIs
API planning isn't just about technical specifications—it's about building something that others will love to use. It's like designing a house where every room serves a purpose and connects logically to others.
Key Questions to Answer:
- Who are the consumers? (Frontend developers, third-party partners, etc.)
- What operations does it support? (CRUD operations, integrations, etc.)
- How will it be secured? (Authentication, rate limiting, etc.)
The Art of Planning
Think of API planning like painting a masterpiece:
- Code-First is like painting without a sketch
- Design-First is like planning your composition
The Code-First Approach
The code-first approach involves jumping straight into coding and creating functionality before documenting or designing the API structure. When I started building APIs, I was a code-first enthusiast. Here's what I learned:
// Day 1: "This seems simple enough!"
app.get('/users', getUsers);
// Week 2: "Oh wait, I need filtering..."
app.get('/users', authenticateUser, validateQuery, getUsers);
// Month 3: "Maybe I should have planned this better..."
Quick Tip ✨: Code-first can work well for prototypes, but document your decisions as you go!
How It Works
- Start with backend development and models.
- Build API endpoints around your database structure.
- Document the API after implementation.
Advantages
- Faster prototyping: Ideal for small teams or personal projects.
- Direct implementation: Focuses on building functionality without upfront planning.
Challenges
- Inconsistent design: APIs may lack uniformity if multiple developers are involved.
- Difficult iteration: Major changes can be costly after development.
The Design-First Approach
The design-first approach emphasizes planning and defining the API’s structure before writing any code. It keeps everyone on the same page. After agreeing upon an API definition, Stakeholders(e.g., testers, and technical writers) can work in parallel with developers.
How It Works
- Use tools like Swagger/OpenAPI to design the API schema.
- Define endpoints, request/response formats, and validations.
- Share the design with stakeholders for feedback.
- Start development once the design is finalized.
Advantages
- Collaboration: Promotes early feedback from stakeholders.
- Consistency: Ensures uniformity across endpoints.
- Mock APIs: Allows frontend teams to begin integration earlier using mocked responses.
Challenges
- Upfront effort: Initial design takes time.
- Requires expertise: Developers must be familiar with design tools and best practices.
Code-First vs Design-First: A Comparison
Code-First
- Speed: Faster for simple projects.
- Collaborations: Limited during the initial stages.
- Consistency: This may vary across endpoints.
- Flexibility: Easy for solo development.
- Scalability: This can become challenging to scale.
Design-First
- Speed: Slower due to upfront planning.
- Collaborations: Encourages early team collaboration.
- Consistency: Ensures a standardized design.
- Flexibility: Great for teams or public APIs.
- Scalability: Designed with scalability in mind.
How to Choose the Right Approach
Choose Code-First If:
- You’re building a quick proof-of-concept or internal API.
- The API consumer is a single, small team.
- You prioritize speed over design.
Choose Design-First If:
- Your API is for external consumers or multiple teams.
- Collaboration and consistency are priorities.
- You’re building a public or long-term API
Practical Steps to Plan Your API
Step 1: Define Your API’s Purpose
Before diving into endpoints and methods, answer these fundamental questions:
- What problem does your API solve?
- Who are your target users?
- What core functionality must you provide?
- What are your non-functional requirements?
Example Purpose Statement:
This API enables e-commerce platforms to manage inventory across multiple warehouses in real time,
ensuring accurate stock levels and preventing overselling.
Step 2: Identify Core Resources
Think of resources as the nouns in your API. For our e-commerce example:
Primary Resources:
- Products
- Inventory
- Warehouses
- Stock Movements
Resource Relationships:
Product
└── Inventory
└── Warehouse
└── Stock Movements
Step 3: Define Operations
Now consider what actions (verbs) users need to perform on these resources:
Products:
GET /products - List products
GET /products/{id} - Get product details
POST /products - Create new product
PUT /products/{id} - Update product
DELETE /products/{id} - Remove product
Inventory:
GET /inventory/{productId} - Get current stock levels
POST /inventory/{productId}/adjust - Adjust stock quantity
GET /inventory/low-stock - List low-stock items
Step 4: Plan Data Models
Define clear, consistent data structures:
{
"product": {
"id": "string",
"name": "string",
"sku": "string",
"description": "string",
"price": {
"amount": "number",
"currency": "string"
},
"inventory": {
"total": "number",
"warehouses": [
{
"id": "string",
"quantity": "number",
"location": "string"
}
]
}
}
}
Step 5: Plan Authentication and Security
Consider security from the start:
- Authentication methods
- Authorization levels
- Rate limiting
- Data encryption
- Input validation
Step 6: Document your API
Create comprehensive documentation:
API Overview
- Purpose and scope
- Getting Started guide
- Authentication details
Endpoint Documentation
- Resource descriptions
- Request/response formats
- Example calls
- Error handling
Use Cases
- Common scenarios
- Integration examples
- Best practices
Conclusion
Both the code-first and design-first approaches are valuable in API development. The key is to choose the one that aligns with your project's needs, team size, and long-term goals. Ultimately, whether you opt for a code-first or design-first approach, the aim is to create an API that developers enjoy using. Sometimes, the journey is less important than the destination, but having a good map can make the trip easier!
Looking Ahead: CollabSphere Case Study
In our upcoming blog series, we'll put these principles into practice by building CollabSphere, a real-time chat system. You'll see firsthand how I transform a code-first project into a design-first masterpiece.
Preview of What's Coming:
- Designing the chat API from scratch
- Creating comprehensive API documentation
- Implementing real-time features
- Handling authentication and security
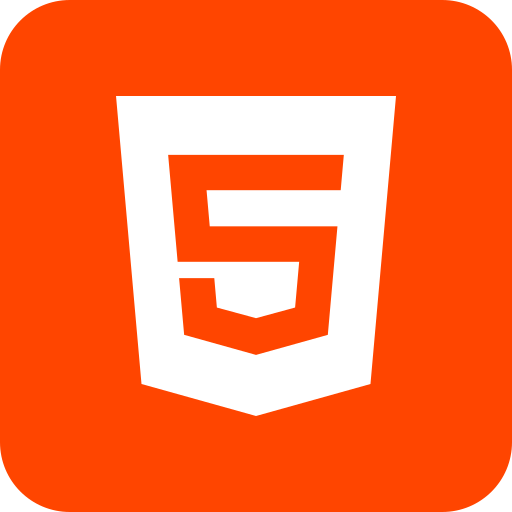
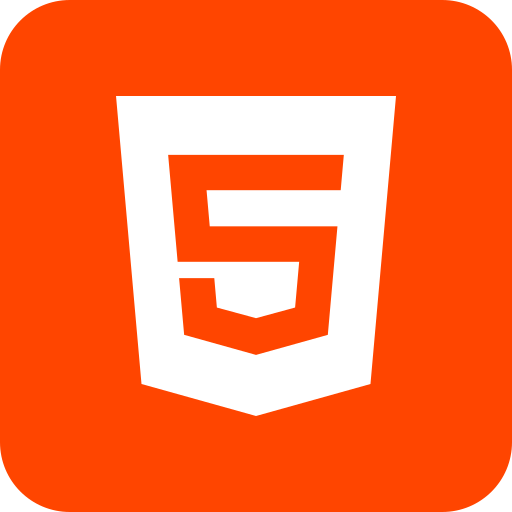
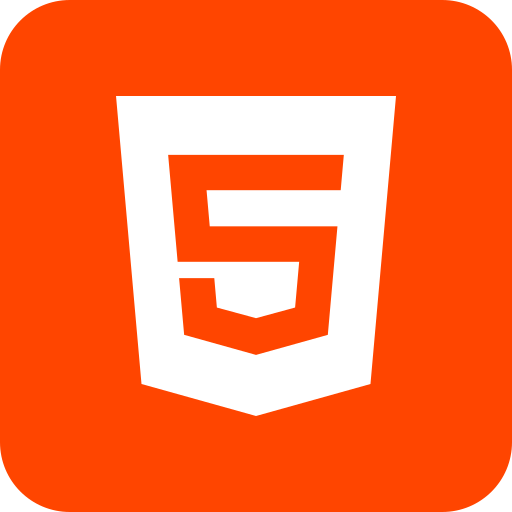
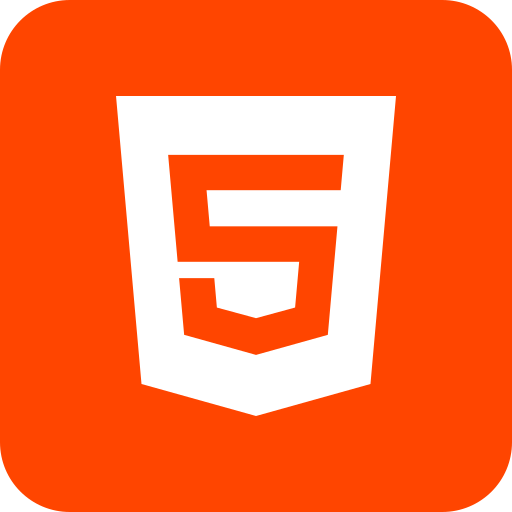
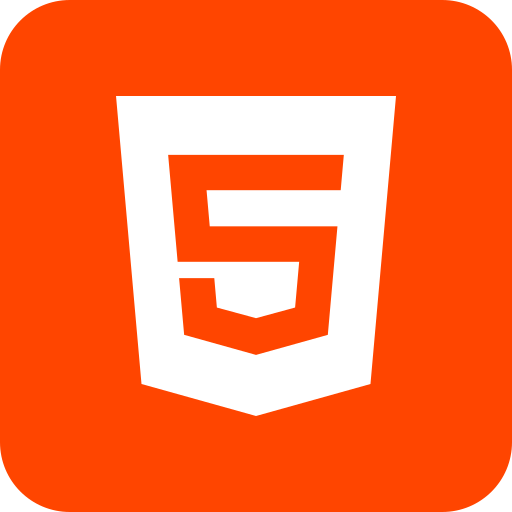
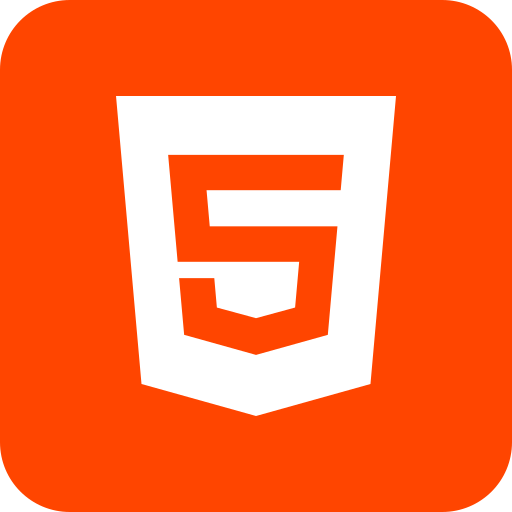
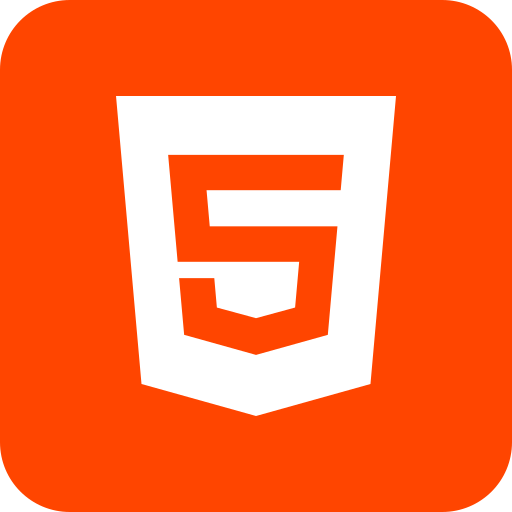
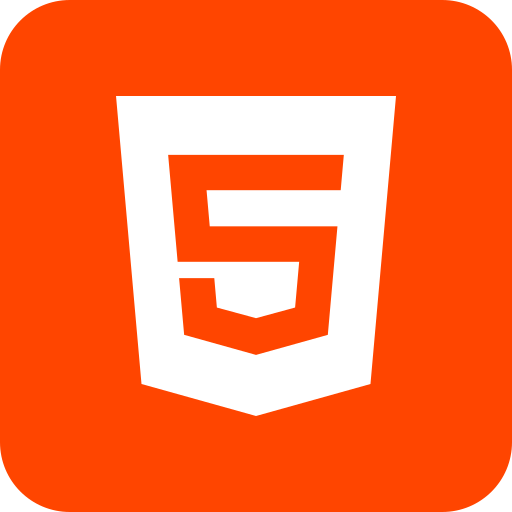
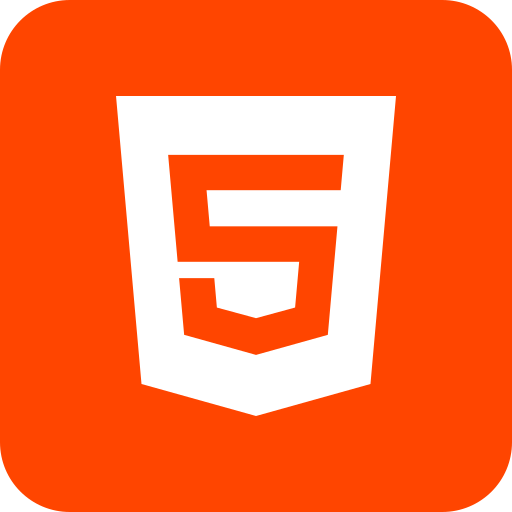
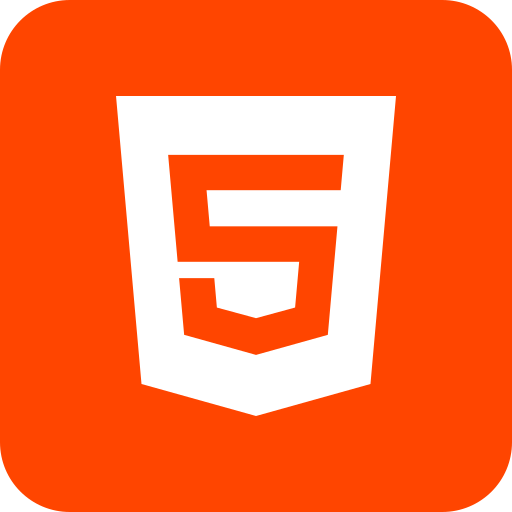
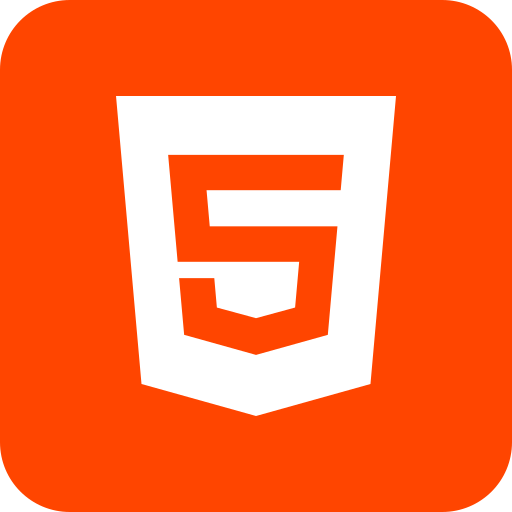
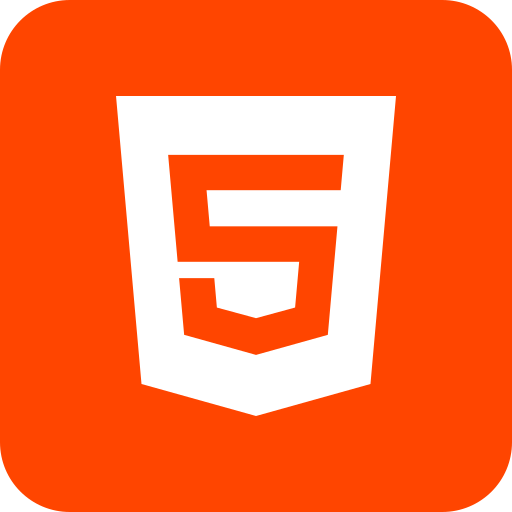
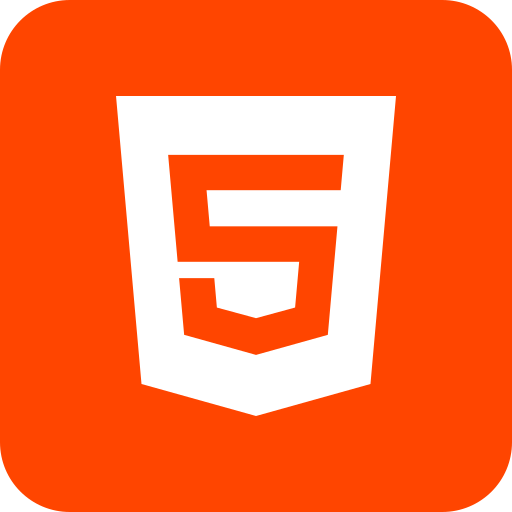
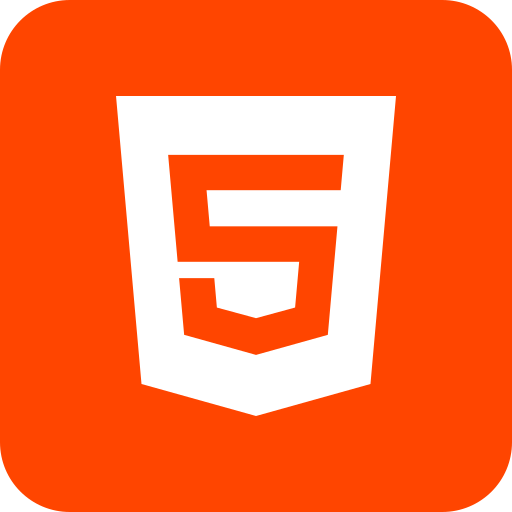
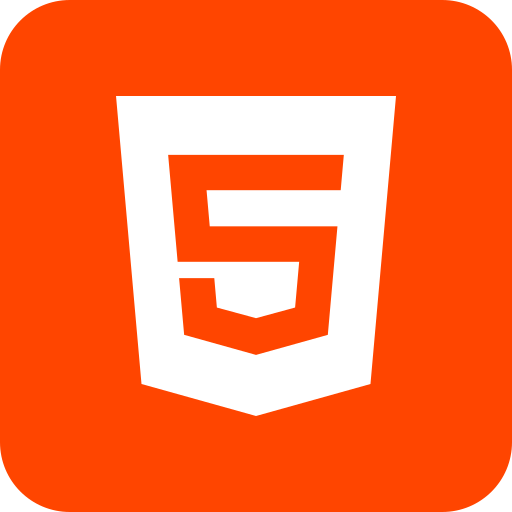
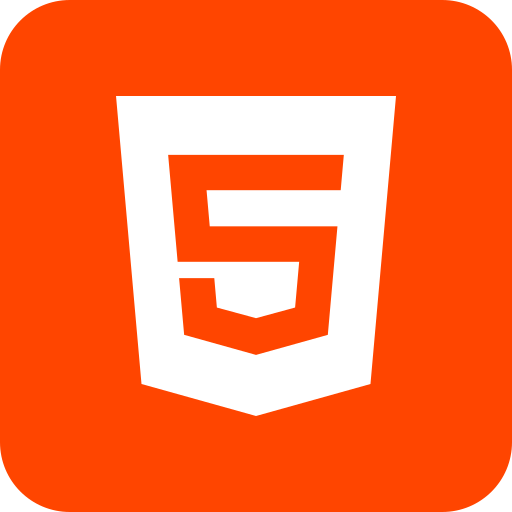
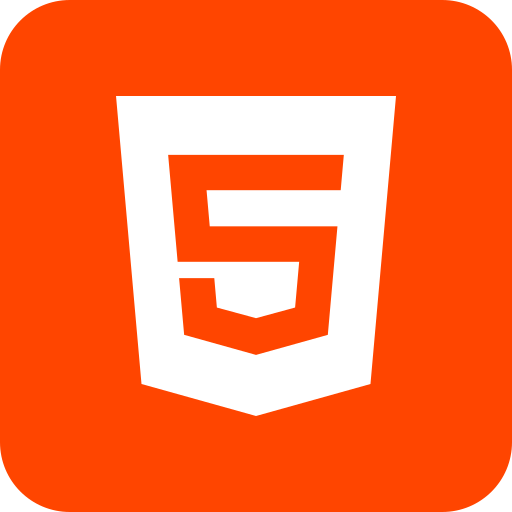
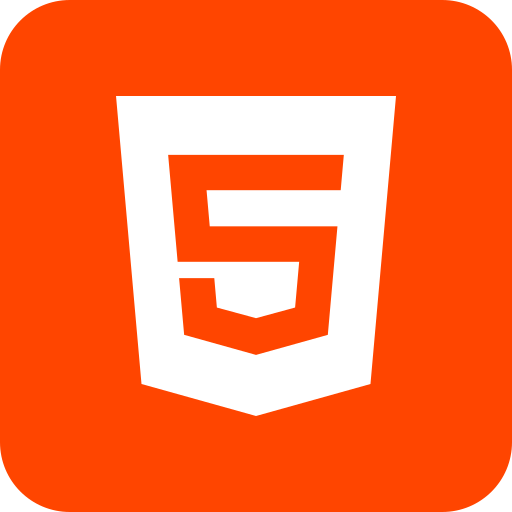
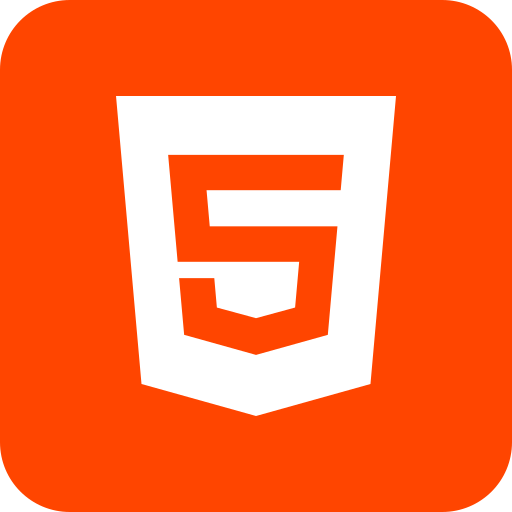
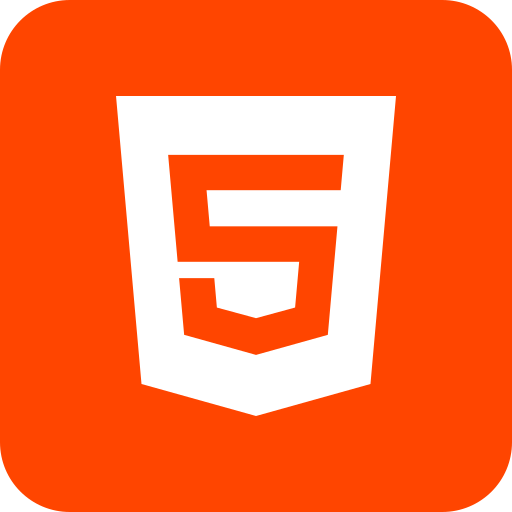
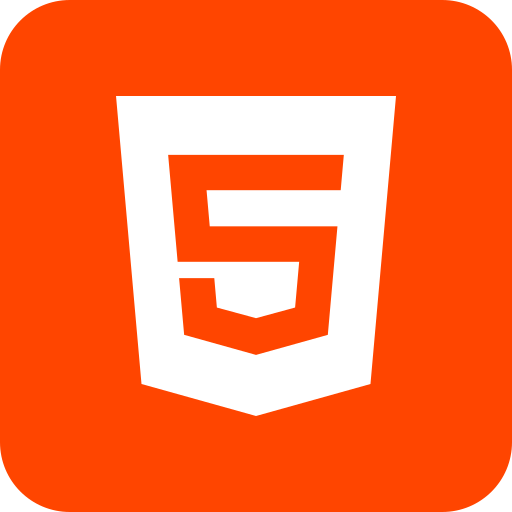
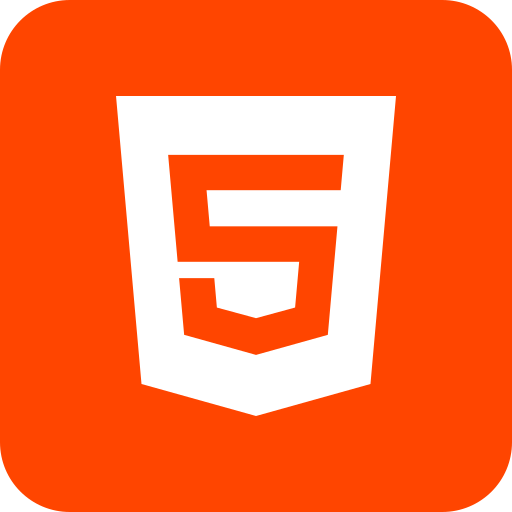
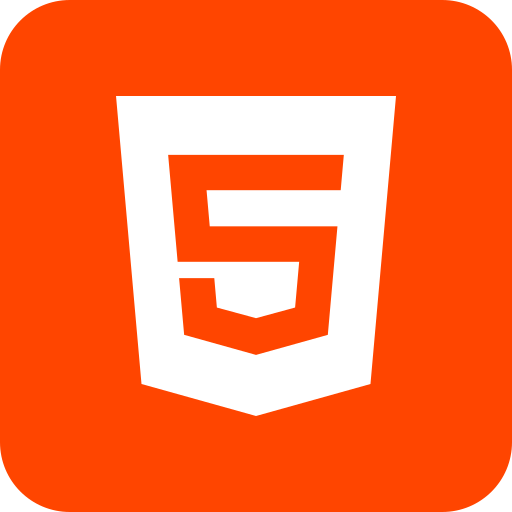
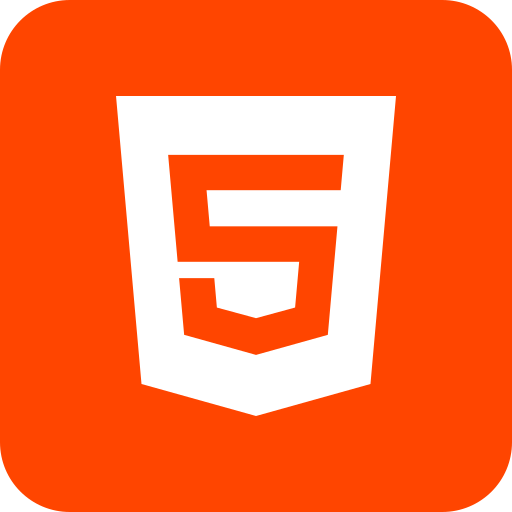
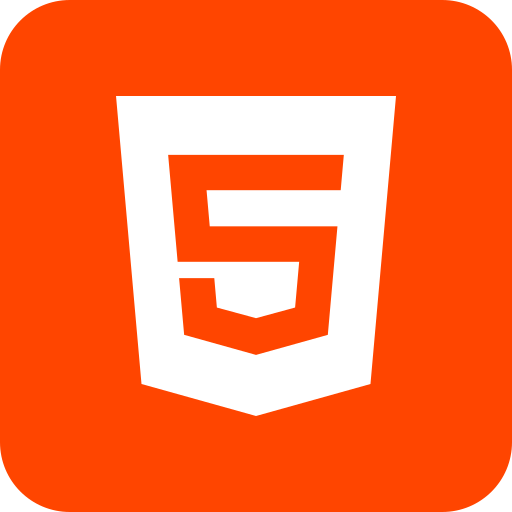
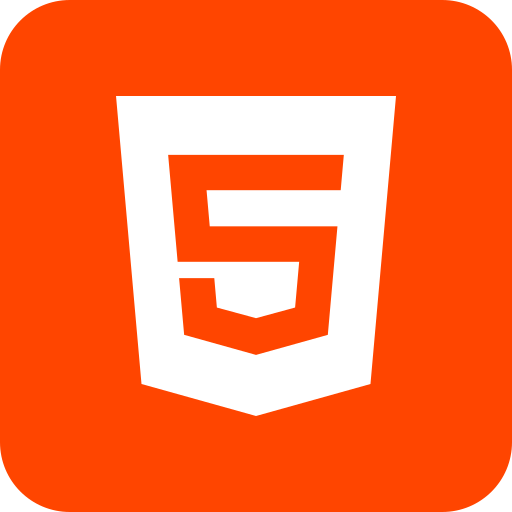
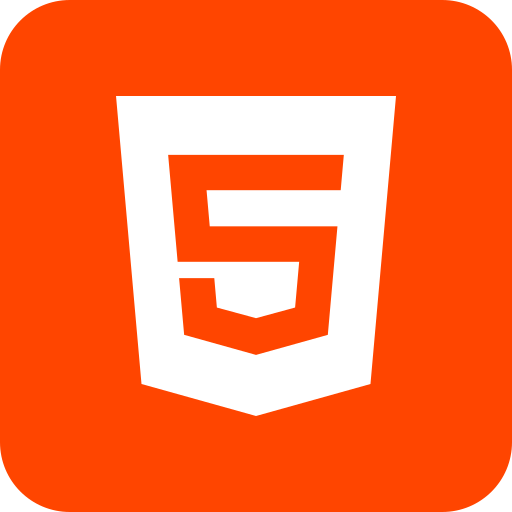
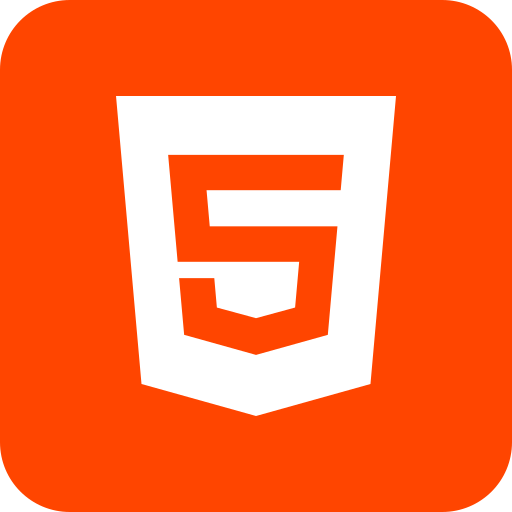
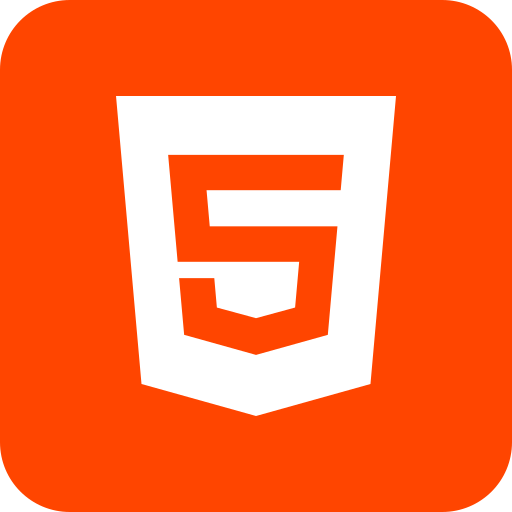
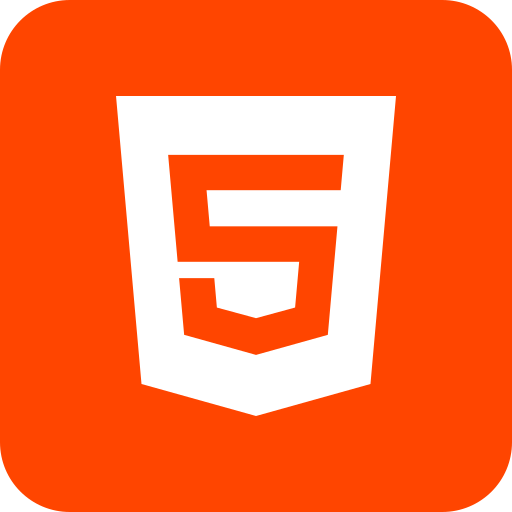
Featured ones: