
dev-resources.site
for different kinds of informations.
Programming Paradigms: Finding Your Development Philosophy
Every aspect of your lifeâyour work, daily routines, and even your thoughtsâis guided by paradigms, whether you realize it or not. A paradigm is simply a pattern or framework that shapes how you see and interact with the world. In this article, when you see the word 'paradigm,' think of it as a pattern.
In programming, paradigms play a similar role. They are proven approaches or thought frameworks used to solve problems effectively. While all programming languages can solve problems, each comes with its own paradigmsâpatterns you can leverage. Mastering these paradigms is key to becoming a great programmer.
Connecting Programming Paradigms to Your Developer Philosophy
Programming paradigms are more than technical toolsâthey reflect deeper philosophical approaches to problem-solving and solution design. Gaining a deep understanding of these paradigms is essential for shaping your mindset and enhancing your effectiveness as a developer.
Example 1: JavaScript's Paradigms and the JavaScript Developer's Mindset
JavaScript is a uniquely versatile language, supporting multiple paradigms, such as procedural, object-oriented, and functional programming. These paradigms aren't just coding techniquesâthey influence how developers think and approach problems.
For example, consider handling a list of user objects to filter out users over a certain age. A procedural approach in JavaScript might involve using for loops and manual condition checks:
const users = [{ name: "Alice", age: 25 }, { name: "Bob", age: 35 }];
const filteredUsers = [];
for (let i = 0; i < users.length; i++) {
if (users[i].age <= 30) {
filteredUsers.push(users[i]);
}
}
console.log(filteredUsers);
This approach reflects a step-by-step problem-solving mindset, where the developer explicitly controls each part of the logic.
Now, consider the functional paradigmâs approach
const users = [{ name: "Alice", age: 25 }, { name: "Bob", age: 35 }];
const filteredUsers = users.filter(user => user.age <= 30);
console.log(filteredUsers);
This method encourages a declarative mindset, focusing on what should happen rather than how to make it happen. By leveraging JavaScriptâs higher-order functions, the developer can write concise, expressive, and reusable code.
For a JavaScript developer, mastering these paradigms shapes their philosophy. A procedural mindset helps break down problems into detailed steps, useful for complex, low-level logic. The functional approach fosters readability and composability, critical for large-scale applications.
This flexibility is both a strength and a responsibility for JavaScript developers. Choosing the right paradigm for the task enhances both their effectiveness and their ability to adapt to diverse challenges, ultimately shaping a versatile and thoughtful developer philosophy.
Example 2: Crossing the Language Divide From JavaScript to Java and Back
For developers moving between Java and JavaScript, the journey reveals fascinating contrasts in programming philosophy. Java's rigid structure and strict typing provide a safety net of rules and predictability. JavaScript, with its dynamic typing and functional features, opens up new creative possibilities - though this freedom requires careful attention to avoid subtle bugs and type-related issues.
The path works both ways: JavaScript developers diving into Java might initially feel restricted by at its strict requirements, but often come to value how the compiler catches errors early and enforces clean design. Java developers exploring JavaScript can leverage their disciplined mindset while discovering more flexible ways to solve problems.
These different approaches ultimately enrich both sides. Java's emphasis on structure can help tame JavaScript's flexibility, while JavaScript's adaptability can inspire more elegant solutions in Java. The result is developers who can thoughtfully blend both paradigms, choosing the right tool and approach for each unique challenge.
The Two Core Programming Paradigms
Programming paradigms are built on two fundamental approaches. From these core philosophies emerge several distinct paradigms, each providing unique methods for addressing programming challenges. The two foundational approaches are:
1. Imperative Programming: The 'How' Approach
Focuses on how to execute tasks step-by-step,like giving detailed directions to a destination. The programmer specifies exactly how to accomplish each task, controlling the program's state and flow.
Here's a more detailed look at its characteristics, principles, and examples:
Core Concepts:
1. State and Mutation
Programs operate on state, which can be changed through assignments, loops, and conditional statements. The state of variables or data structures can be modified during program execution.
2. Control Flow
Uses control structures like loops (for, while), conditionals (if-else), and sometimes goto statements to dictate the flow of execution. This contrasts with declarative approaches where control flow might be implicit.
3. Procedures and Functions
While imperative programming can involve functions, they are often used to group statements that perform operations on data, changing its state or producing side effects.
4. Sequential Execution
Instructions are generally executed one after another, following the sequence laid out in the code, unless altered by control structures or function calls.
5. Side Effects
Operations that modify global or external state, like writing to files, modifying global variables, or changing the display, are common in imperative programming.
Characteristics:
1. Explicit Instructions
You tell the computer exactly what to do, step-by-step. This can make the code very readable for simple tasks but can become complex for larger systems.
2. Modularity
Through procedures or functions, code can be broken down into modular pieces, though without the encapsulation benefits of OOP.
3. Memory Management
Often requires manual memory management in lower-level languages (like C), though higher-level imperative languages might include garbage collection.
4. Variables
Used to store and update data throughout the program's execution.
Types of Imperative programming
Imperative programming encompasses all programming approaches that explicitly describe how a program operates through sequences of commands that change state. Its main subcategories are:
1. Procedural Programming
- Emphasizes structured sequences of commands/procedures
- Focuses on step-by-step execution
- Examples: C, FORTRAN
2. Object-Oriented Programming (OOP)
- Based on objects containing data and methods
- Core concepts: encapsulation, inheritance, polymorphism
- Examples: Java, C++
3. Structured Programming
- Uses control structures (loops, conditionals)
- Avoids GOTO statements
- Often overlaps with procedural programming
4. Modular Programming
- Emphasizes separation of functionality into modules
- Focuses on code organization and reusability
- Often used alongside other paradigms
5. Event-Driven Programming
- Program flow determined by events (user actions, messages)
- Common in GUI and reactive applications
- Examples: JavaScript for web applications
6. Concurrent/Parallel Programming
- Handles multiple operations simultaneously
- Includes both threading and distributed processing
- Examples: Go, Erlang's imperative features
7. Command-Based Programming
- Based on discrete commands that modify state
- Often used in command-line interfaces and automation
- Examples: Shell scripting
Example in C
Here's an example of imperative programming in C, showcasing state modification through a simple loop:
#include <stdio.h>
int main() {
int sum = 0; // Initialize state
for (int i = 1; i <= 10; i++) { // Control flow with a loop
sum += i; // Mutate state
}
printf("Sum of numbers from 1 to 10 is: %d\n", sum); // Side effect: output to console
return 0;
}
Advantages:
1. Performance
For tasks requiring direct hardware manipulation or when performance is critical, imperative programming can be more efficient.
2. Control
Gives you fine-grained control over what's happening in your program, which can be beneficial in system programming or when dealing with hardware.
3. Familiarity
Many programmers start with imperative techniques, making it an intuitive way to think about problem-solving in computing.
Disadvantages
1. Complexity
As programs grow, imperative code can become harder to manage due to the complexity of maintaining state, especially in multi-threaded environments.
2. Debugging
Since state can change frequently, identifying where and why an error occurs can be challenging.
3. Less Reusability
Without the abstractions of OOP or functional paradigms, code reusability can be less straightforward.
These categories often overlap and complement each other. For instance, a modern application might use OOP principles within a modular structure, handle events, and implement concurrent operations.
Popular Languages
- C: A classic example of imperative programming with direct memory manipulation capabilities.
- C++: While it supports OOP, much of its core remains imperative, especially for performance-critical code.
- Java: Although an OOP language, its imperative roots are evident in how methods operate on objects' states.
- Python: Supports imperative style alongside others; many Python scripts are written imperatively.
- Bash/Shell Scripting: Imperative by nature, focusing on executing commands in sequence.
2. Declarative Programming: The 'What' Approach
Describes the desired outcome rather than the steps to achieve it, like ordering a meal without specifying how to cook it. The programmer focuses on the end result, letting the underlying system determine the execution details.
Core Concepts:
1. Focus on Outcome
You describe the result or the properties of the solution without detailing the steps to achieve that result.
2. Abstraction of Control Flow
The control flow is abstracted away from the programmer. The system or language runtime decides the execution path to meet the declared conditions or goals.
3. High-Level Specifications
Declarative languages often provide high-level abstractions that allow developers to work at a level closer to the problem domain rather than the machine level.
4.Logic Over Control
The emphasis is on specifying the logic (rules, facts, constraints) that should hold true rather than on controlling the flow of the program.
Types of Declarative Programming:
1. Functional Programming
- Based on mathematical functions
- Avoids state changes and mutable data
- Examples: Haskell, Erlang, Clojure, Ocaml
2. Logic Programming
- Based on formal logic
- Programs express facts and rules
- Examples: Prolog, Mercury
3. Database Programming
- SQL and similar query languages
- Describes what data to retrieve/manipulate
- Focuses on data relationships
Characteristics
1. Immutability
Many declarative paradigms encourage or enforce immutability, reducing side effects and making programs more predictable.
2. Parallelism
Since there's less explicit control over how things are done, operations can often be parallelized more easily by the system.
3. Maintainability
By focusing on what rather than how, code can be easier to maintain and understand, especially for complex systems where the "how" might change without affecting the "what".
4. Less Error-Prone
With less manual management of state and flow control, there's potentially less room for errors like off-by-one mistakes or state corruption.
Examples:
- SQL
SELECT name, age FROM employees WHERE department = 'IT';
Here, you're declaring what data you want, not how to fetch it from the database.
- HTML:
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
You declare the structure you want, not the steps to render it.
- Prolog (Logic Programming):
parent(alice, bob).
parent(alice, charlie).
grandparent(X, Y) :- parent(X, Z), parent(Z, Y).
You state facts and rules, and Prolog determines how to find grandparents.
- Functional Programming in JavaScript (using React):
const List = ({ items }) => (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
You describe what the UI should look like based on the data without detailing how to update the DOM.
Benefits:
1. Expressiveness
Can lead to more concise, readable code that describes intentions clearly.
2. Flexibility
The same declarative specification can often be implemented in multiple ways by the system, allowing for optimizations without changing the source code.
3. Scalability
Easier to scale due to the inherent parallelism and the abstraction from low-level details.
4. Testability
Declarative code can be easier to test since it's more about specifying outcomes than managing states.
Challenges:
1. Learning Curve:
The shift from imperative to declarative thinking can be challenging, especially for those accustomed to detailed control over execution.
2. Performance
In some cases, declarative approaches might introduce overhead if the system's method of achieving the goal isn't optimal for all scenarios.
3. Debugging
Can be less straightforward since the system determines the "how", potentially making it harder to trace execution steps.
Conclusion
In practice, many modern programming environments blend declarative and imperative styles, leveraging the strengths of each. Declarative programming shines in areas where the focus is on data or where the problem can be described more naturally in terms of what rather than how.
Why Programming Paradigms Matter
If you aim to be among the best programmers, you should care about programming paradigms because they:
1. Build your problem-solving arsenal
They equip you with a versatile toolkit to tackle a wide range of programming challenges. This flexibility enables you to choose the most effective approach for any given problem. By mastering when and how to apply each paradigm, youâll create more efficient, elegant solutions across various domains and levels of complexity.
Each paradigm offers unique tools and perspectives. For instance, functional programming shines in concurrent systems and data processing pipelines. Object-oriented programming excels at modeling complex domains with many interacting parts..
2. Improve Code Quality and Design:
As a self-taught developer, I wasn't initially exposed to programming paradigms because I didn't follow a structured curriculum like in university. Instead, I learned by diving into projects and figuring things out on the job.
I vividly recall an experience early in my career as a junior developer. I was assigned a challenging task that needed to be implemented in PHP using CodeIgniter. To complete it, I wrote a single class containing multiple procedures (donât worry about the terminologyâweâll explore it later). While the solution worked, the class became excessively long and cluttered.
During the code review, a senior engineer refactored my class, breaking it down into specialized functions, each handling a specific responsibility. The result was clean, organized, and far easier to understand. I was amazed by the transformation and realized how much I had to learn. That experience inspired me to dive deeper into PHP and master Object-Oriented Programming (OOP).
3. Enhance Understanding Of Legacy and Modern Systems
As a developer, youâll often encounter legacy systemsâcode written by others that you need to maintain, refactor, or even rebuild. If youâre not familiar with different programming paradigms, understanding such codebases can be challenging. However, a solid grasp of paradigms will help you recognize patterns in the code, making it easier to navigate, interpret, and improve. Modern systems are also rarely built using a single paradigm.Take React's component model, which combines functional programming concepts with declarative UI design.Understanding these intersections is crucial for building sophisticated software.
4. Improve Communication and Collaboration
Common Language: Understanding programming paradigms gives you a shared vocabulary for discussing concepts with peers. This is especially valuable in collaborative environments where team members may have diverse programming backgrounds.
Mentorship and Teaching: You may take on the role of mentoring or teaching others. A strong grasp of paradigms enables you to clearly explain why specific approaches are more effective, helping others deepen their understanding of programming principles.
5. Unlock Innovation, Research Opportunities, and Advanced Career Growth
Mastering programming paradigms fuels innovation by enabling you to adapt, combine, or even create new approaches to problem-solving. Many high-level roles, such as research positions, software architects, AI/ML engineers, high-performance computing, game development or technical leaders, demand a deep understanding of diverse methodologies. This knowledge not only helps you specialize effectively but also keeps your skills sharp and your mind engaged, paving the way for continuous growth and preventing career stagnation.
Stay tuned for our upcoming deep dives into each imperative and declarative programming paradigm, where we'll explore real-world applications, key concepts, and practical examples. Join us as we unravel the unique strengths and use cases of each approach.
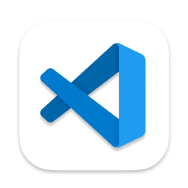
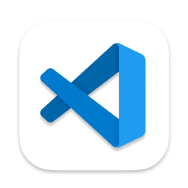
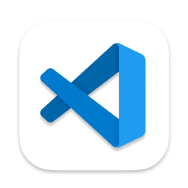
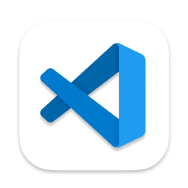
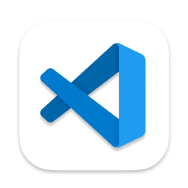
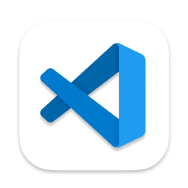
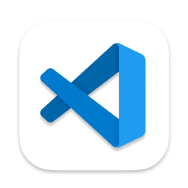
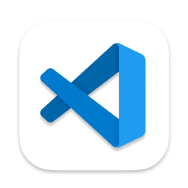
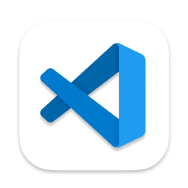
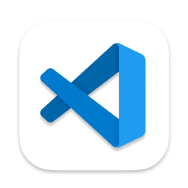
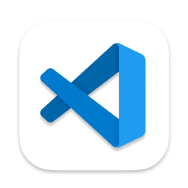
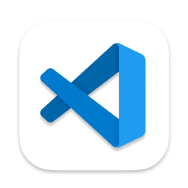
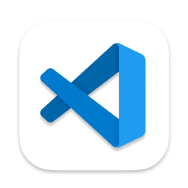
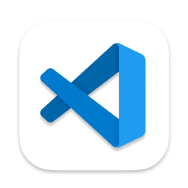
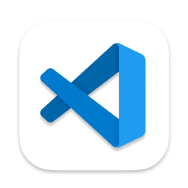
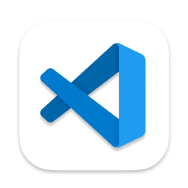
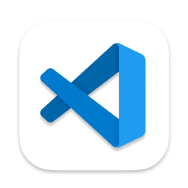
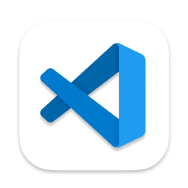
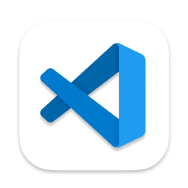
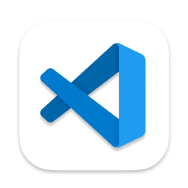
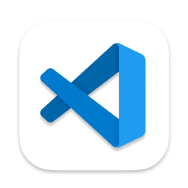
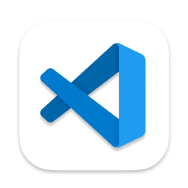
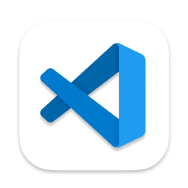
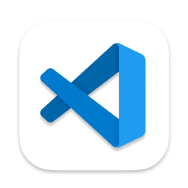
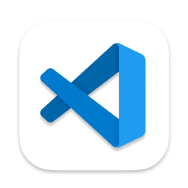
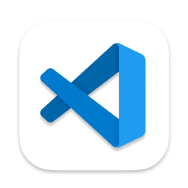
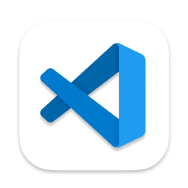
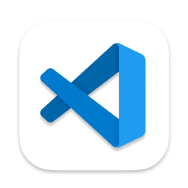
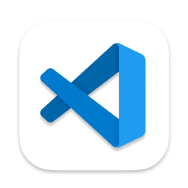
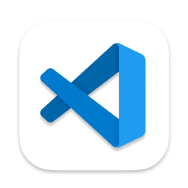
Featured ones: